
Loop assistance
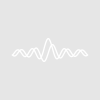
Hello! I am finalizing my code and noticed an issue. I am using BinarySearch to find an ending for my curve. When the value is out of the bounds, BinarySearch returns a -2. I want to make a loop that prompts the use for a new ending point. If the value makes BinarySearch return a -2, then I want the loop to start over. If the value makes BinarySearch return anything over 0, then I want the program to print the result and end the loop. Unfortunately, I have tried many methods to get this to work but to no avail.
Any assistance would be appreciated.
variable index_value = BinarySearch(selection_fit, y_half) if (index_value >= 0) // if index_value is greater than or equal to 0, then break loop print " index value = ", index_value else if (index_value == -2) do if (index_value > 2) break endif DoAlert /T="ERROR: STOP POINT OUT OF RANGE", 0, "The stop point entered is out of range for the half-life. Click 'OK' and select a stop point within the range" Prompt stop_point, "Enter desired stop point" // prompt user to give new stop value DoPrompt "Enter_Stop_Point", stop_point CurveFit/M=2/W=0 dblexp_XOffset, selection [50,stop_point]/X=Time__seconds_[50,stop_point]/D while (index_value > 0) // continue from break #1 print " index value = ", index_value break endif
An issue I keep encountering is that after the error, the new index_value is not saved and only the -2 is registered. I think if this issue was resolved, the rest of the code would work
1. check your while() condition. If you want always to exit the loop via the break at the start, you can use while(1), otherwise while(index_value<=0)
2. your code does not assign a new value to index_value after the curvefit. Perhaps you need another BinarySearch to prevent the loop from running forever.
3. enable the debugger, set a breakpoint and step through your code to see what is happening.
July 23, 2022 at 12:32 am - Permalink
In reply to 1. check your while()… by tony
you might also want to consider what happens if curvefit fails, or if the user enters a problematic value for stop_point. programming to catch fitting errors is a bit more tricky.
July 25, 2022 at 12:43 am - Permalink
In reply to 1. check your while()… by tony
Thank you for such a quick response! I tried using the code you sent but it was affecting the values for my other variables. I'm not sure why. I took elements of your code and tried it in my program to see if that could resolve the issue. However, when I did, I encountered the same issue of index_value being read as -2. Using debugger, I discovered that the values for the new graph were really the old wave values from the first curve-fitting. I corrected this by using KillWaves to get rid of the values from the first wave, allowing the new wave with the curve-fitting values to be used. The old curve wave and the new curve wave have the same name. That's why I was having so many issues. Thanks for your help!
July 25, 2022 at 08:28 am - Permalink