
2D function: doublesidedexp vs lor
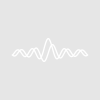
gzvitiello
I'm trying to develop a custom fitting function to make a 2D data fitting. On the x axis I need to fit a double sided exponential and on the y axis I need to fit a lorentzian. I would like to make a function that would be able to fit as many points as I need, therefore the initial guesses would be based on N (x,y) coordinates where N is the number of peaks. So far I have developed this code for a single (x,y) value and I would like to make it for N peaks:
#pragma rtGlobals=1 // Use modern global access method.
Function twosidedExpXlor(w,x,y) : FitFunc
Wave w
Variable x
Variable y
//CurveFitDialog/ These comments were created by the Curve Fitting dialog. Altering them will
//CurveFitDialog/ make the function less convenient to work with in the Curve Fitting dialog.
//CurveFitDialog/ Equation:
//CurveFitDialog/ f(x,y) = L0 + A*exp(-abs(x-x0)/tau)*1/((y-y0)^2 + B)
//CurveFitDialog/ End of Equation
//CurveFitDialog/ Independent Variables 2
//CurveFitDialog/ x
//CurveFitDialog/ y
//CurveFitDialog/ Coefficients 6
//CurveFitDialog/ w[0] = L0
//CurveFitDialog/ w[1] = A
//CurveFitDialog/ w[2] = x0
//CurveFitDialog/ w[3] = tau
//CurveFitDialog/ w[4] = y0
//CurveFitDialog/ w[5] = B
return w[0] + w[1]*exp(-abs(x-w[2])/w[3])*1/((y-w[4])^2 + w[5])
End
I also would like to develop a way to find the peaks. I have heard that the SARFIA would do this since I'm using a tiff image to load the data. But how do I create a 3D wave from a tiff image file?
Thanks in advance,
Gabriel
You can use the size of the w coefficient wave to compute the number of peaks to fit. Something like this (warning: I'm writing this code directly into the edit box in the browser, it's not tested in any way):
Note that I have eliminated the special comments, which are now incorrect and irrelevant. When you write a fit function like this, you make it impossible for the Curve Fit dialog to parse the code and figure out how many coefficients to fit. Indeed, the number may vary from one time to another. So if you use the Curve Fit dialog, you will have to pre-make an appropriate coefficient wave with the right number of points for the number of peaks you want to fit.
If SARFIA will do it for you, then by all means use it. Finding peaks can be very tricky and you could spend a lot of time developing the code. If SARFIA doesn't work out, I have done some work for customers using ImageAnalyzeParticles as the basis for a peak finder. It requires peaks that don't have much overlap, and it works best on low-noise data.
Try the ImageLoad operation.
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
December 2, 2011 at 09:48 am - Permalink
May I declare the number of peaks in the begining of the procedure file so it will atomaticaly generate the function, then find the peaks and fit them? If the awnser is yes how do I do that? I would like to plot on Gizmo the fitting results afterwards.
I apreciate a lot your help, sorry for the silly questions but I'm new to this.
Thank you
December 2, 2011 at 10:29 am - Permalink