
Appending Data to Existing Wave
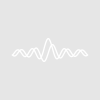
astrotool
Is there anyway I can append the files together so I don't have a bunch of segmented data?
Searching IGOR's Help only gives me options to append to graphs not to waves.
If you're just asking how to append points to a wave, you can use either the Redimension or InsertPoints operations to add additional points onto a wave. You could then re-save the entire wave to your data file, overwriting the file in the process.
If overwriting the data file every hour is too time consuming or otherwise not a good solution, you could create a new wave each hour in your acquisition program, and append each successive wave to your data file every hour. To avoid the need to overwrite your data file every time you save to it, you'll want to either save each new wave in an unpacked experiment or save your data file as a type that can be appended to. Igor text files can be appended. For my data acquisition, I use the HDF5 XOP included with Igor to add new waves to a HDF5 file that I save my data into.
When you then open your data file, if you want all points to be in one single wave, it's easy to combine all of your waves into a single wave for analysis. You can do this in a loop, or the Concatenate operation will possibly be of use to you.
May 22, 2008 at 06:50 am - Permalink
I have 3 columns of data with about 3600 points each, per file.
I want to append one file to another so I then have
3 columns of data with 7200 points each, then 1080 points each and so on as I add on the data from other files
May 22, 2008 at 09:51 am - Permalink
So, as an example, you can try something like this:
Does this help?
May 22, 2008 at 12:08 pm - Permalink
I uploaded the two actual data files I am using. The only columns I am concerned about really are Time, Current SS, and CCN Count Number
I just want to make these two files into one basically. That way I can analyze the data for the day, not just per hour.
Eventually I will want to take like 24 of these and put them into one, but figuring out how to join 2 of them together is what I am trying for
May 22, 2008 at 01:03 pm - Permalink
Create a function to load a file into a wave ...
Create a function to add waves together into CatWave ...
Run these two in a loop function ...
HTH
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
May 22, 2008 at 01:47 pm - Permalink
Obviously the second function is easier but you may learn about wave indexing from the first.
May 22, 2008 at 01:51 pm - Permalink
May 22, 2008 at 02:49 pm - Permalink
May 22, 2008 at 04:12 pm - Permalink
I took that code and modified it into this to work for me how I needed it
May 23, 2008 at 02:28 pm - Permalink
You could also do ...
... to cover for cases where wave0 ... wave47 might skip something.
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
May 23, 2008 at 02:31 pm - Permalink