
assigning a variable through an if elseif statement
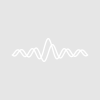
jasond7
Mon, 10/17/2016 - 02:18 pm
Make seq1st3rd={1,3,3},seq1st5th={1,5,5},seq1st7th={1,7,7},seq1st9th={1,9,9},seq1st11th={1,11,11}//Waves to find end of 1st harmonic
FindSequence /V=seq1st3rd Harmonic
Variable/G/C trans1st3rd=V_Value
FindSequence /V=seq1st5th Harmonic
Variable/G/C trans1st5th=V_Value
FindSequence /V=seq1st7th Harmonic
Variable/G/C trans1st7th=V_Value
FindSequence /V=seq1st9th Harmonic
Variable/G/C trans1st9th=V_Value
FindSequence /V=seq1st11th Harmonic
Variable/G/C trans1st11th=V_Value
if (trans1st3rd!=-1)
Variable/G/C end1st=trans1st3rd
elseif (trans1st5th!=-1)
Variable/G/C end1st=trans1st5th
elseif (trans1st7th!=-1)
Variable/G/C end1st=trans1st7th
elseif (trans1st9th!=-1)
Variable/G/C end1st=trans1st9th
elseif (trans1st11th!=-1)
Variable/G/C end1st=trans1st11th
else
Variable/G end1st=DimSize(Harmonic,0)
endif
FindSequence /V=seq1st3rd Harmonic
Variable/G/C trans1st3rd=V_Value
FindSequence /V=seq1st5th Harmonic
Variable/G/C trans1st5th=V_Value
FindSequence /V=seq1st7th Harmonic
Variable/G/C trans1st7th=V_Value
FindSequence /V=seq1st9th Harmonic
Variable/G/C trans1st9th=V_Value
FindSequence /V=seq1st11th Harmonic
Variable/G/C trans1st11th=V_Value
if (trans1st3rd!=-1)
Variable/G/C end1st=trans1st3rd
elseif (trans1st5th!=-1)
Variable/G/C end1st=trans1st5th
elseif (trans1st7th!=-1)
Variable/G/C end1st=trans1st7th
elseif (trans1st9th!=-1)
Variable/G/C end1st=trans1st9th
elseif (trans1st11th!=-1)
Variable/G/C end1st=trans1st11th
else
Variable/G end1st=DimSize(Harmonic,0)
endif
This code compiles fine if I omit the last portion:
else
Variable/G end1st=DimSize(Harmonic,0)
Variable/G end1st=DimSize(Harmonic,0)
That part is there to basically say if you don't find any transitions from value 1 to another value, then the original wave must only have values of 1, so just assign the variable end1st to whatever the dimension size of that wave is. Without this last piece, the procedure won't work for waves that only have values of 1. The error that I get is that it says that the variable end1st already exists. So I'm confused as to why all the previous elseif statements work properly (no variable seems to be assigned unless one of the if statements is true) but I get the compile error when I try to use that last bit. Not sure if it matters but I've also tried the following to replace the last else statement but have the same problem with the variable already being in use.
elseif (trans1st3rd==-1)&&(trans1st5th==-1)&&(trans1st7th==-1)&&(trans1st9th==-1)&&(trans1st11th==-1)
Variable/G end1st=DimSize(Harmonic,0)
Variable/G end1st=DimSize(Harmonic,0)
Thanks in advance for any help!
You might be happy with the Extract command, which doesn't preserve the original wave dimension but at least counts the number of values for you?
The /INDX flag may also be useful so that you know where those occurred in the original sequence.
The command "SelectNumber" could also work...for example:
HarmonicFives=SelectNumber(Harmonic[p]==5,NaN,Harmonic[p])
This will result in a wave filled with 5's where the original wave had 5's but NaN everywhere else.
Another option is MatrixOp with the "equal" command, but it fills the output wave non-matching values with 0's instead of NaN's in the above example, and its output wave type may not be what you need.
If you need to reduce the size of the waves output (e.g. to get rid of the 0's or NaN's), you could follow up with a Sort and Redimension.
October 17, 2016 at 03:34 pm - Permalink
- First, I don't know why you use the /C flag (complex variable), as the output is clearly not complex. Ist there some reason to use complex values later in the procedure?
- Also, there is no /C flag on your last statement, which should rather give you an "inconsistent type" compile error. Maybe you use an older Igor version ... (which one?)
- I would recommend to avoid using global variables in general until you are really really sure you need them. Just declare a normal variable once and assign the values in your if statement (you could also first assign a normal variable and then save the result in a global variable in a separate step later if needed).
Hope that helps a bit with future coding endeavors.
October 17, 2016 at 11:06 pm - Permalink
October 18, 2016 at 06:45 am - Permalink
FindSequence/V=seq1st3rd Harmonic
if (V_value!=-1)
end1ist = v_value
break
endif
FindSequence/V=seq1st5th Harmonic
if (V_value!=-1)
end1st = v_value
break
endif
...
while(1)
OR
for (ic=0;ic<5;ic+=1)
seqwave = seqmatrix[ic][p]
FindSequence/V=seqwave Harmonic
if (v_value!=-1)
end1ist = v_value
break
endif
endfor
The first uses the seq waves as you have. The second collapses the seq waves in to a matrix (that you have to set up) and then iterates using the matrix.
Caveat: These still have to be tested (they are "off the top of my head" if you will).
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
October 18, 2016 at 09:58 am - Permalink
To debug your code you rather should use the build-in debugger, which gives you insight into the values of variables while the function is running (and all kinds of other information) instead of just the last (global) value after everything is done. Execute the following line in the Igor command window to learn more about the debugger:
DisplayHelpTopic "The Debugger"
October 19, 2016 at 09:19 pm - Permalink