
Batch curve fitting and muti-peak fitting
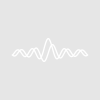
lingxiaoabc
I have a problem to do curve fitting peak fitting for a typical peak from Raman spectrum. I want to fit the peak with 2 Gaussian functions, that is y=y0 + y1*exp(-((x-x1)/w1)^2)+y2*exp(-((x-x2)/w2)^2).
I use multi-peak fit 2.0 to deal with single data manually, and then use the batch curve fitting to fit the other datas using the result from the multi-peak fit 2.0 as initial guess. I can easily get the fitting results in terms of the parameters y0, (peak1) y1,x1,w1, (peak2)y2,x2,w2. However I want to know the parameters of the Guass peak, i.e the location, Height, Area, FWHM of peak1 and peak2, which is not shown in the results. How to fix this?
Thanks you.
SetDataFolder $( saveDF+BatchDFName)
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
April 30, 2014 at 04:52 pm - Permalink