
Calculating Percentiles of 1 wave grouped based on another wave
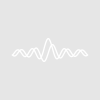
rhoover
Hello!
I am using Igor 9.02 and am not great at programming so thought I would ask here for advice.
I have a list of points that have an associated age (modeled_cr) and an associated unit type (Unit). I am trying to calculate the 10th percentile of modeled_cr values based on the Unit. I tried to use the percentiles and box plot function but I can only get percentiles based on the entire list, not divided up by unit. Is it possible to separate them out? I am attaching a subset of my data as a text file.
Thanks in advance for any help!
Rachael
You can use the Extract operation to create individual waves with a given string from the Unit wave. For instance, I created a wave of the values corresponding to "A29" like this:
This could be encapsulated into a user-defined function to make it easier to use.
January 3, 2024 at 04:11 pm - Permalink
In reply to You can use the Extract… by johnweeks
Okay, is there a way to easily create individual waves for all unique Unit labels? In my full dataset I have ~300 different Unit types.
January 3, 2024 at 04:42 pm - Permalink
I think this will do what you want. I've basically put John's code into a loop.
January 3, 2024 at 05:13 pm - Permalink
In reply to I think this will do what… by Ben Murphy-Baum
Thanks, Ben. I am getting an error when I try to run the function (command error: expected wave name, variable name or operation). Is that an issue with how I am loading in my data?
January 3, 2024 at 05:35 pm - Permalink
Make sure you're providing the function with valid waves. To be safe, provide the full path to each wave. Running this from the command line might look like:
extractUnitData(root:Unit, root:modeled_cr)
where root:Unit is a text wave with the units, and root:modeled_cr is a numeric wave. This is assuming you loaded each column of your text file into a separate wave, of course.
January 3, 2024 at 05:42 pm - Permalink
In reply to Make sure you're providing… by Ben Murphy-Baum
Okay I am having an issue with loading in my data as waves, so I will figure that out and then get back to this. Thanks for the help!
January 3, 2024 at 07:07 pm - Permalink
In reply to Make sure you're providing… by Ben Murphy-Baum
I got this working and have individual waves of modeled_cr for each unique unit. What would be the best way to calculate the 10th percentile for each wave?
January 3, 2024 at 08:01 pm - Permalink
This function:
will make two waves: a text wave with the names of the waves contained in wlist, and a numeric wave with the tenth percentile value from each of the waves in the list. The tenth percentile is determined in unsophisticated way: it simply gives you the interpolated value corresponding to the position one tenth of the way from the smallest to largest value in the sorted values.
I used Ben's function to extract the individual waves, then invoked my function like this:
quantiles(WaveList("modeled_cr_*", ";", ""))
You may wish to think whether this is truly what you want :)
January 4, 2024 at 09:57 am - Permalink
In reply to This function: Function… by johnweeks
Great, thank you so much for your help! I really appreciate it!
January 4, 2024 at 03:06 pm - Permalink
Building off the code you all provided I tried to write additional code to use the Percentile function to loop through each new unique wave name and calculate the 10th percentile. I am getting an error for this line -
that says expected comma, and if i added a comma it says "expected string variable or string function." Any ideas whats going on?
Thanks!
January 4, 2024 at 04:19 pm - Permalink
DisplayHelpTopic "WaveList"
Running that from the command line will bring up the documentation for the WaveList function. Note that it expects 3 parameters: the matchStr, a separatorStr, and an optionStr. Your code is missing the last two parameters.
For future reference in the documentation - any parameter enclosed in square brackets is an optional parameter, everything else is required.
January 4, 2024 at 04:24 pm - Permalink
Additionally, there is another mistake in your code and a detail you might want to reconsider:
First, you might notice that waveName is colored in orange. This is because 'WaveName' is the name of a builtin operation. While such code works in principle, I cannot recommend reusing names for builtin function or operations as string / variable name as this may lead to confusion and possibly bugs down the road.
The second line will not work. You cannot just equate a string and a wave reference in this way. You would need to use the '$' reference operator. Read more about this here:
DisplayHelpTopic "Converting a String into a Reference Using $"
January 4, 2024 at 07:31 pm - Permalink
In reply to Additionally, there is… by chozo
Thanks for the info, I just had this realization this morning ha
My current issue is that uniqueWaveList is returning one string, is there an easy way to split this string up, or is there an alternate function that does it for me?
January 5, 2024 at 09:45 am - Permalink
It looks like you want the ListToTextWave() function.
January 5, 2024 at 10:58 am - Permalink