
Can't get rid of the error "Ambiguous wave point number"
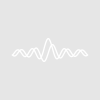
jgladh
Hi,
I have got a problem when a call a function from another function. The function I'm calling should return a wave, but I only get the error massage "Ambiguous wave point number". I don't really understand whats wrong because the called function is a Function/WAVE, function. Somehow I can see where the problem is so if anyone have an idea it would be much appreciated.
Best
Jörgen
Call function
Function/WAVE get_BG_slope(theYwave, theXwave, from, to) Wave theYwave, theXwave Variable from, to Make/O/N=(2,2) A Make/O/N=(2) B Variable i A[0][0] = theXwave[from] A[0][1] = 1 A[1][0] = theXwave[to] A[1][1] = 1 B[0] = theYwave[from] B[1] = theYwave[to] MatrixInverse/O A MatrixOp/O C = A x B Make/O/N=(numpnts(theXwave)) line for(i=0;i<numpnts(theXwave);i+=1) line[i] = theXwave[i]*C[0]+C[1] endfor return line End
Main function
Function finalWaves(theOffWave, theOnWave, theXwave, endnumber) Wave theOffWave, theOnWave, theXwave Variable endnumber String finWaveName, offWNameShift Variable i, OffBase, OnBase Variable to = numpnts(theXwave)-2 Variable from = 1 finWaveName = "finalSiganl_"+NameOfWave(theOffWave)[strsearch(NameOfWave(theOffWave),"_",0),strlen(NameOfWave(theOffWave))]+"norm" offWNameShift = NameOfWave(theOffWave) + "shift" CurveFit/Q/H="00"/TBOX=768 line theOffWave /X=theXwave /D Wave W_coef OffBase = W_coef[0] CurveFit/Q/H="01"/TBOX=768 line theOnWave[0,endnumber] /X=theXwave /D OnBase = W_coef[0] Make/O/N=(numpnts(theOnWave)) $offWNameShift Wave w = $offWNameShift w = theOnWave w -= (OnBase-OffBase) Make/O/N=(numpnts(theOnWave)) $finWaveName Wave ww = $finWaveName ww = (w-theOffWave)/theOffWave Make/O/N=(numpnts(theOnWave)) driftLine driftLine = 0 driftLine = get_BG_slope(ww, theXwave, from, to) End
I think the error is the last line of your main function. You make driftline, set it to 0 and then you need to get the wave back from your wave function and then assign it to driftline, rather than what you have written there which is giving an error because you are getting a wave rather than a point value or range of values. So I think this would work (untested)
Alternatively, since your main function ends there, you could edit the /WAVE Function to make the wave named driftline rather than line and make it a regular function. Then you can just write get_BG_slope(ww,theXwave.from, to) in your main function instead of those final 3 lines.
April 1, 2021 at 02:34 pm - Permalink
In reply to I think the error is the… by sjr51
Thank you so much it worked excellent now
Best
Jörgen
April 1, 2021 at 02:39 pm - Permalink
The solution you have so far creates two output waves. line is created by get_BG_slope and driftLine is created by finalWaves. You probably want only one output wave.
A simple way to do that is to have get_BG_slope create the driftLine wave and create no wave in finalWaves. In that case, I would rename get_BG_slope with a name that better describes what it does, such as CreateBGSlopeOutputWave.
You could make CreateBGSlopeOutputWave more general by not hardcoding the name of the output wave in the function but rather by passing the output wave name from the higher level function. For example:
You do not need the "WAVE wOut =" part in the second function unless you want to do something with the output wave after it is returned from CreateBGSlopeOutputWave. But leaving it there does no harm either.
April 6, 2021 at 02:31 pm - Permalink
Just some other thoughts to tidy up.
Is the for loop really needed instead of this?
Would it help to use FREE waves in place of the make/O in those cases where you don't need the waves (e.g. A and B and C).
April 6, 2021 at 03:12 pm - Permalink
Hi jjweimer,
no probably not but somehow an old habit .
best
Jörgen
April 6, 2021 at 03:26 pm - Permalink
In reply to The solution you have so far… by hrodstein
Thank you for that input hrodstein
Best
Jörgen
April 6, 2021 at 03:27 pm - Permalink