
Checking for blank cells?
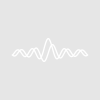
Hi all,
Two waves TopWave and BotWave were meant to simultaneously store collected data. In a perfect world, the two waves would match up. Indices with blank cells will be the same for both waves, and cells with data will be the same. I could then simply add the waves together and histogram.
Because the data collection is imperfect, the blank cells and data do not match at the same indices. This means wave 1 has events that wave 2 is missing, and where wave 1 and wave 2 both have numbers, I double count events.
In the code below, I'm trying to fill a 1D wave by checking if Topwave has a number, and Botwave is blank cell. I'll put only Topwave events into a new wave, and do the same for botwave. Where there are overlapping events, aka numbers in the same index for both topwave and botwave, I'll arbitrarily pick Topwave or BotWave events and generate a 3rd wave that turns all the double counted events into single events.
My question is: how do you check if the cells are blank? I don't think they're 0's. Someone suggested I could check the length of the cell by casting it into a string and check for NaN if I tell Igor that I'm expecting a float.
Thanks in advance. I'm new to coding
Function TopBot(TopWave, BotWave) Wave TopWave, BotWave Variable i, N=numpnts(TopWave) Make/O/N=(N) OverlapHist, TopHist, BotHist for (i=0; i<N; i+=1) if ((TopWave[i] != NaN) && (BotWave[i] == NaN)) TopHist[TopWave] = TopHist[TopWave]+1 endif if ((BotWave[i] !=NaN) && (TopWave[i] == NaN)) BotHist[BotWave] = BotHist[BotWave]+1 endif if ((TopWave[i] !=0) && (BotWave[i] !=0)) OverlapHist[TopWave] = OverlapHist[TopWave]+1 endif endfor end
Hi, It's not possible to check NaN in that way. You need to do
if ((numtype(TopWave[i]) == 2) && (numtype(BotWave[i]) == 2))
Your wave assignments are also written incorrectly. TopHist[TopWave] makes no sense because TopWave is a wave and not an integer (same applies to the RHS).
Rather than write this in a loop, you can also probably do this by conditional wave assignment.
August 21, 2018 at 12:41 pm - Permalink
In reply to Hi, It's not possible to… by sjr51
Does that mean a blank cell is exactly referred to as the number 2? What happens when I want to conditionally check for the number 2?
Would it make more sense if I did TopHist[TopWave][p], I had the impression that it was wave[rows][columns] but because it's a 1D wave I shouldn't have columns.
When you say conditional wave assignment do you mean
Sorry, I'm not familiar with what you mean by conditional wave assignment.
August 21, 2018 at 12:56 pm - Permalink
NaN is not a number. This means you can't test it using == or !=. By definition it doesn't equal or not equal anything. The way to test it is to test its number type.
DisplayHelpTopic "numtype"
As I understand it you want to put 1 in the index of the wave TopHist if your condition is met? You can do this with
TopHist[i] = 1
As I said, you just need an integer in the square brackets.
A conditional wave assignment would be something like:
TopHist[] = (NumType(TopWave[p]) != 2) && (NumType(BotWave[p]) == 2) ? 1 : 0
This doesn't need to be in a loop, because it assigns each row of TopHist for all values of p. Check out the help topic for an explanation of how this works.
August 21, 2018 at 01:16 pm - Permalink
In reply to NaN is not a number. This… by sjr51
I updated the code to what you suggested, but it didn't seem to work for the 0th index. (I attached the output waves)
What do I look up in displayhelptopic to learn more about conditional wave assignment? Does p go all the way to the Nth row? Does this not work if TopWave and BotWave have different numpnts? How do I control the boundaries? and why is this preferential to a loop? When I say it in plain language: I want to go through TopWave and BotWave to check for NaN, from i=0 to i=N. For me, it seems that the most straightforward method is a loop.
Sorry for all the questions, this has been super helpful I really appreciate it!
August 21, 2018 at 01:55 pm - Permalink
The reason row 0 doesn't work is that you have the extra line TopHist[i] = 1. The variable i initialises at 0 so you are changing that row after doing the correct assignment. I've confused you a bit with suggesting two solutions.
Try this:
p.s. please don't edit your OP - my replies don't make sense if you change the code that I'm commenting on. These threads are useful for people reading in the future trying to figure out the same thing.
August 21, 2018 at 02:20 pm - Permalink