
Condensing Code for Similar Functions
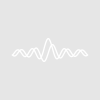
Ken
CheckBox Series,pos={151,70},size={64,14},proc=CheckProc,title="Series 1"
CheckBox Series,fStyle=17,variable= V
Function CheckProc(ctrlName, x) : CheckBoxControl
String ctrlName
Variable x
Another_function ()
End
So for example,
CheckBox Series_1,pos={151,70},size={64,14},proc=CheckProc_1,title="Series 1"
CheckBox Series_1,fStyle=17,variable= V_1
CheckBox Series_2,pos={151,95},size={64,14},proc=CheckProc_2,title="Series 2"
CheckBox Series_2,fStyle=17,variable= V_2
...
Function CheckProc_1(ctrlName, x) : CheckBoxControl
String ctrlName
Variable x
Another_function ()
End
Function CheckProc_2(ctrlName, x) : CheckBoxControl
String ctrlName
Variable x
Another_function ()
End
...
Many thanks!
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAHuntsville
May 26, 2011 at 09:39 am - Permalink
May 31, 2011 at 09:44 am - Permalink
It makes a panel and condenses the creation of as many checkboxes as you like in a loop. Checkboxes and their control variables share common base names with a numeric extension.
In this example a single, very simple example procedure is called when the boxes are "clicked".
May 31, 2011 at 01:57 pm - Permalink
(EDIT)
Oh. My apologies. Now I understand. You want to have a code create a panel for you. For that, use the code by jtigor, however the procedure name can just be CheckProc with the above code to automatically call depending on check box name.
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAHuntsville
May 31, 2011 at 03:18 pm - Permalink
You *do* have to have a different action procedure for different types of controls because they each carry different kinds of information.
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
June 1, 2011 at 09:30 am - Permalink
June 10, 2011 at 10:19 am - Permalink