
Conversion to hexadecimal
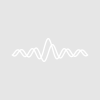
neogin
Tue, 07/13/2010 - 06:09 am
My current issue is that I need to convert decimal number into hexadecimal and then split the resulting hex number in to. An example :
I have v=10000
I want vHex to equal v value in hex, so VHex=2710
and then I need a wave like this : WaveHex={0x27,0x10}
For conversion :
I tried sscanf it works but only work with string ( sscanf "10000","%x",vHex )
I tried printf, it's better : it works with variable but I don't seem to be able to get the result store in another variable( printf "%x", v)
I still have no idea how to split the result to get the final wave.
thanks for your help,
best,
Gautier
I don't understand what you mean by:
Assuming that WaveHex is a numeric wave, this is identical to:
The only difference is that, in the first case, you are expressing the value in hexadecimal and in the second case you are expressing it in decimal. The value stored in the wave is identical in both cases.
Perhaps what you really want to do is to split a 16-bit value into two 8-bit values. For example:
Edit out
ModifyTable ModifyTable format(out)=10,sigDigits(out)=2
If you really do want to convert a 16-bit unsigned number into two strings that represent the high and low byte in hex:
Variable num // Assumed to be an unsigned 16-bit value
String text
sprintf text, "%4x", num
return text
End
Function Demo()
String text = NumToHex(10000)
String low = text[2,3]
low = "0x" + low
String high = text[0,1]
high = "0x" + high
Print high, low
End
July 13, 2010 at 10:38 pm - Permalink
To give you the big picture, I need to send a string of hex number, right now I'm using that :
VDTWriteBinaryWave2 temp
The issue is that it is static, and I need to be able to change these values dynamically.
The sentence is in fact a command (code 0x06) and tree numbers, here 0 0 32000, so 0x00,0x00,0x00,0x00,0x7D,0x00. The two central numbers are entered by the user in decimal and the soft must create the adequate wave and send it via VDTWriteBinaryWave2.
it is clearer to you ?
best,
gautier
G.
July 15, 2010 at 01:55 am - Permalink
VDTWriteBinaryWave2 temp
This does not send anything as hex. It sends it as binary. You are entering the values to send in hex but that is irrelevant. You could just as well enter 6 instead of 0x06 or 125 instead of 0x7D. Either way, it is converted to unsigned 8-bit binary and stored in your unsigned 8-bit binary wave. VDTWriteBinaryWave2 sends the contents of the wave as binary. It does not matter how you entered the data in the wave.
In other words, no matter how you get data into temp, whether as a hex number, as a decimal number, using a wave assignment statement or whatever, VDTWriteBinaryWave2 does the same thing - it sends 8-bit binary bytes.
July 15, 2010 at 01:04 pm - Permalink
Variable num // 16bit valeur
String text
sprintf text, "%4x", num
return text
End
Function HighBit(num)
variable num
variable a
String text = NumToHex(num)
String high = text[0,1]
sscanf high, "%x",a
return a
End
Thanks for your help
July 16, 2010 at 02:48 am - Permalink
What you wanted to do was extract the high byte of a two-byte integer value. This has nothing to do with hexadecimal. My expression could just as well be written:
July 16, 2010 at 10:59 am - Permalink