
curve fitting and constraints
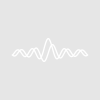
masheroz
I think that the best way to do so is to fit a curve subject to an additional constraint that the value of the curve must be less than or equal to the data at every point.
The constraints make it easy to constrain the value of the coefficients, but I can't see how to further constrain based on the actual data values.
In Excel, I could add a column for the result of (data - calculated background) and have a single cell equal to the minimum of that column, and constrain the model such that the cell value is greater than or equal to zero.
.
Question: How can I constrain the model such that the model is always less than the data?
http://www.igorexchange.com/node/6006
In outline the process is as follows:
1. Fit your quadratic (or whatever function you desire) and calculate residuals.
2. Determine the data points that have the largest (positive) values for their residuals and mask these from subsequent fits.
3. Repeat 1 and 2 a few times.
The parameters are the number/fraction of points you exclude at each iteration, and the number of iterations.
The fit will then end up being on the background only (if the data is well behaved).
Note: there is a chance that a quadratic fit actually fits the peak initially - this is more likely to happen if there is more peak than background in your data. In this case, I suggest you make the first iteration a straight line fit.
HTH,
Kurt
March 17, 2015 at 12:21 am - Permalink
I'll have a look.
March 17, 2015 at 12:42 am - Permalink
Code to remove background from a 1 dimensional wave:
code to apply the 1D case to a 2D wave of 1D waves that I need to fit:
March 17, 2015 at 10:13 pm - Permalink
I think the following should work (not tested):
March 18, 2015 at 12:58 am - Permalink
Oh, and also this should work (not tested):
March 18, 2015 at 01:06 am - Permalink