
DAQ question from a complete novice
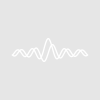
Matt Carnie
I've been using Igor Pro now for a few months for DAQ but only using the built in panels in NIDAQ tools mx. I bit the bullet last week and decided I needed to start programming (holy cow, this is tricky!). I'm a complete novice to programming full stop, so I've bought myself a few C books to learn at home in the evenings. I'm using my hardware/software to conduct measurements on dye solar cells and have written a few very simple functions to turn lights on and off, flash LED pulses etc using the DAQmx_CTR_OutputPulse function to control a switching transistor (I'm new to electronics too! phew!)
The first simple programme (or so I thought) I wanted write was is to do a "photovoltage decay experiment". Very simply, I illuminate my solar cell for 15s whilst measuring the OCP with DAQ analogue input. After 15s the light goes off and you measure the voltage dropping to zero (this can be up to a minute in some devices).
This is what I have so far:
To turn the light on for 15s and then off I have this:
Function RunPVDecay()
String DeviceName = StringFromList(0, fDAQmx_DeviceNames())
DAQmx_CTR_OutputPulse /DEV=DeviceName /DELY=0 /FREQ = {1000, 0.5} /IDLE=0 /NPLS=15000 /RATE=-1 /STRT=1 /OUT=("PFI1") 1;
End
and that works fine.
I've also managed to write a few lines to capture the analogue input and covert it into a wave.
Function GetVOC()
String DeviceName = StringFromList(0, fDAQmx_DeviceNames())
Wave VOC
DAQmx_CTR_OutputPulse /DEV=DeviceName /DELY=0 /FREQ = {1000, 0.5} /IDLE=0 /NPLS=15000 /RATE=-1 /STRT=1 /OUT=("PFI1") 1;
VOC=fDAQmx_ReadChan (DeviceName, 4, -1, 1,0)
Display VOC
modifygraph live=1
setaxis left -0.8, 0
End
This also works ok but what I really want to do (and here is my question finally!) is when the user pushes the button to run the function on the panel that I have built, I want a prompt to come up asking for the wave name, I want that wave to be saved in a certain folder and to be returned immediately to a graph and then all further waves to be appended to the same graph.
I feel a bit cheeky asking for help and maybe I should go away and learn to code properly first! But if anybody out there does want to help, I would be extremely grateful.
Many many thanks,
Matt
learning programming can be a daunting task at first, but it will reward you well whatever field you're in. Good luck on your journey!
I have a function that does something similar to what you want. Basically, you provide it with a data folder the wave will be created in (can be any valid DFREF), and it will ask the user to specify an output name. Then it will check whether the name is valid and unique, and will keep prompting the user until both are satisfied. If the user cancels, the function will return an empty string, otherwise it will return the desired wave name. Here is the function:
To use this function, embed it in your code as follows:
As for making it so that the code runs when the user presses a button, that's not included. I'd suggest you try it yourself first and then just post back here if you can't get it to work.
July 17, 2012 at 12:03 am - Permalink
I love this forum. Perhaps in around 10 years or so I might be able to contribute my own codings.
Thanks again,
Matt
-----------Update-----------------------
It works! I have a few more things to work out but I should go and figure them out for myself.
Many thanks,
Matt
July 20, 2012 at 01:35 am - Permalink