
Draw on-top of Button control
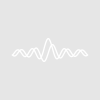
gregorK
Is it possible to draw on-top of a button (button control)? I was even thinking of using a CustomControl but it seems that one cannot draw on-top of a generic button ("frame=1" option), or am I mistaken? I know that I can use a custom picture for the buttons but I would prefer to use the generic button because I have already put a lot of effort in a programmatic drawing of specific items in IGOR ... I would be happy for any ideas, solutions for this rather specific issue. Thanks!
Best regards,
Gregor
You say that "I know that I can use a custom picture for the buttons but I would prefer to use the generic button because I have already put a lot of effort in a programmatic drawing of specific items in IGOR". Is this a dynamic, run-time generated picture? If it is something that can be drawn once and saved, then you could make a ProcPicture from it that can be saved with your code and then used as the picture for a button. Draw the picture into a graph or panel window, copy it, go to Misc->Pictures to load it from the clipboard, and click Copy Proc Picture. That puts code into the clipboard that can be pasted into a procedure file.
If you must draw it at runtime, then a custom control is your only option.
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
February 13, 2013 at 09:01 am - Permalink
The Procedure picture (created previously with the drawing tools) is the "button" outline, and the Igor drawing stuff is found in the drawscale( ) function. That is where you could put your own drawing routines. The extra necessary item is the MouseDown event which would call your "button" procedure function in place of my "beep" command. Test this by executing Panel0() from the command line.
February 13, 2013 at 09:55 am - Permalink
John: yes indeed it is a dynamic drawing which can get redrawn (basically part of its color changes) at different times. Clearly if the buttons are drawn on top I will need to use a custom control for this. Could I ask you for a minimal working example? I could not understand from the help file how I can draw at runtime a custom drawing "inside" a custom control.
Thanks for your kind help.
Regards,
Gregor
February 13, 2013 at 12:00 pm - Permalink
--Jim Prouty
Software Engineer, WaveMetrics, Inc.
February 13, 2013 at 12:44 pm - Permalink
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
February 13, 2013 at 12:54 pm - Permalink
The following is an example of my code to illustrate this:
In playing with this I have found some weird behaviour - if you change the coordinates of the rounded rectangle to the following: "DrawRRect 50,50,150,100" the custom control interferes with the picture in that its rectangle is not drawn on any more. I am using Igor 6.30Beta03 on a PC running Windows 7. Has anyone got any ideas about this?
Best regards,
Kurt
February 13, 2013 at 02:06 pm - Permalink
Gregor
February 14, 2013 at 12:40 am - Permalink
I offer this simple example:
--Jim Prouty
Software Engineer, WaveMetrics, Inc.
February 18, 2013 at 11:30 am - Permalink