
Extracting Line Profiles from Image
vmmr5596
I have a set of data that looks according to the attached picture. Each row corresponds to an absorbance spectra. As of right now, I can manually extract individual absorbance profiles using the "Image Line Profile" feature present in Image Processing. However, I would like to automate this process.
This is the procedure I have so far:
Function ExtractAllTimeSpectra(image,tlist) String tlist //List of times of spectra of interest Wave image Variable i Wave spec0 Make/O/N=2048 spec0 for(i=0;i<ItemsInList(tlist);i=i+1) spec0 = image[i][p] String newspec="a_Prof"+num2str(i) Duplicate/O spec0, $(newspec) endfor End
My code currently only produces the very last spectra of the series. Also, the tlist portion does not seem to be working.
Any suggestions?
Thanks!
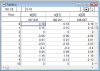
July 27, 2016 at 02:52 pm - Permalink
You'd call it with something like
ExtractAllTimeSpectra(wave0,"0;2;10;")
. I haven't tested the code. I'm not sure if you can name a new wave in MatrixOp like that, you might have to name it something else and then rename or duplicate it.July 27, 2016 at 11:47 pm - Permalink
Your code works fine for me with no modification when I run the following commands in the command window:
Perhaps make sure that you're formatting the "tlist" string properly?
July 28, 2016 at 11:18 am - Permalink
ExtractAllTimeSpectra(testImage,"a;b;d")
you get the same result. tList is not being "read", by the code.July 28, 2016 at 12:05 pm - Permalink
True, tList isn't doing anything useful at this point other than giving the number of spectra to extract. My point was that the original code does indeed extract unique rows from the 2D array and put them in individual waves. The OP said that only the last spectra was being extracted, which is not the case.
July 28, 2016 at 02:02 pm - Permalink
Here's how the working version looks:
July 29, 2016 at 09:41 am - Permalink