
Fixed parameters for optimize
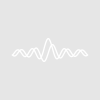
serrano
Hi all,
Is there an easy way to hold parameters when using optimize, like the /H flag of curvefit?
I'd like to do a complex optimization that depends on >10 parameters and would like to allow the user to keep selected parameters fixed. Adding penalties for deviations from the initial guess kind of works, but this doesn't feel very clean to me.
The only way to hold a parameter during Optimize is to move it from the list of X values into the parameter wave. I see the value of being able to do that, since one common use for Optimize is to implement curve fitting by means other than least squares. It would be tricky to implement... I'll think about it.
November 29, 2022 at 11:25 am - Permalink
Thanks, that worked. If anyone is interested, a possible implementation is below.
Quick follow-up question: Is there a way to access the Hessian in case I'd like to implement error estimation?
December 1, 2022 at 06:23 am - Permalink
No, the Hessian is internal to the OPTIF9 package that we didn't write. It's pretty old FORTRAN code in the public domain. The Hessian is in there somewhere...
With a bit of effort you can write a Hessian function using finite differences if necessary. You have already shown ability to put in the effort!
December 1, 2022 at 01:09 pm - Permalink