
FUNCREF to a static function not working?
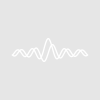
gregorK
In my code I would like to use a function reference. According to the help file the prototype function cannot be static, but the referenced function can be (i.e. one can store a static function into a FUNCREF). But it seems this is not working on Igor 6.37, or am I misunderstanding something from the help file?
Basically I have a function from which I want to call various static functions using a FUNCREF. I wrote a (non-static) prototype function, but then when I create a FUNCREF like
FUNCREF protofunc f = $sFunc
, where sFunc
is a string with the name of the (static) function I would like to call, the FuncRefInfo(f)
function returns a "failed" func reference (i.e. f
is pointing to the prototype function). All the functions are part of the same procedure file.If I remove the
static
keyword from the function declaration (of the function I want to call via a function reference) the function reference works as expected ...Thanks for any help or suggestions.
Gregor
April 24, 2017 at 07:56 am - Permalink
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
April 24, 2017 at 09:11 am - Permalink
Just check the output of the
FuncRefInfo
lines.--
Gregor K
ALOISA Beamline
Elettra Synchrotron
April 24, 2017 at 12:35 pm - Permalink
FUNCREF variables can refer to external functions as well as user-defined functions. However, the prototype function must be a user-defined function and it must not be static.
Although you can store a reference to a static function in a FUNCREF variable, you can not then use that variable with Igor operations that take a function as an input. FuncFit is an example of such an operation.
A.G.
WaveMetrics, Inc.
April 24, 2017 at 01:07 pm - Permalink
Yes, the prototype function in my case is not static.
Here is the issue, I cannot store a reference to a static function at all.
Furthermore, I am not using that reference as an input to an Igor operation.
Therefore according to the help file which I have read the above code example should work.
Can You please clarify what I am misunderstanding (doing wrong) here?
Thanks!
--
Gregor K
ALOISA Beamline
Elettra Synchrotron
April 24, 2017 at 01:42 pm - Permalink
April 24, 2017 at 05:27 pm - Permalink
The #functionName syntax isn't intended, but this is:
--Jim Prouty
Software Engineer, WaveMetrics, Inc.
April 24, 2017 at 06:07 pm - Permalink
Ah ha!
When I am back at my desktop, I'll have to dig up the post from long ago about using Modules and Independent Modules and Statics.
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
April 24, 2017 at 07:24 pm - Permalink
Jim (and others) thank you very much for clarifying. It seems I forgot that the static keyword is bound to the use of Modules.
As always I appreciate your help very much!
Gregor
--
Gregor K
ALOISA Beamline
Elettra Synchrotron
April 25, 2017 at 01:19 am - Permalink
The moduleName pragma is needed only if you refer to a static function either from outside of the procedure file you're using or if you refer to the static function with a FUNCRef.
You can call a static function from within the same procedure file without having a #pragma moduleName=something. Not always needing the pragma is the source of people's confusion.
--Jim Prouty
Software Engineer, WaveMetrics, Inc.
April 25, 2017 at 08:56 am - Permalink
Found it: "Synopsis: Programming With Modules and IndependentModules"
August 6, 2010
http://www.igorexchange.com/node/1688
--Jim Prouty
Software Engineer, WaveMetrics, Inc.
April 25, 2017 at 09:09 am - Permalink
Thanks!
I've added a link there back to this thread as an update.
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
April 26, 2017 at 08:19 am - Permalink