
generating random variable x between [0,1]
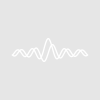
daggaz
random_x = enoise(.49999) + 0.5
which gives me .00001 to .99999, which is close enough for most intents.. Is there a proper way to do this?
random_x = enoise(.49999) + 0.5
August 28, 2013 at 07:51 am - Permalink
I guess what I am asking is if there is hardcoded function random(a,b) that returns a random value x between [a,b] using a flat PDF?
August 28, 2013 at 08:06 am - Permalink
And the answer would be no.
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
August 28, 2013 at 09:26 am - Permalink
Note that the actual probability of getting a random value that is exactly 'a' is vanishingly small.
A.G.
WaveMetrics, Inc.
August 28, 2013 at 10:15 am - Permalink
a: you're probably doing something wrong
b: you will run into other precision and granularity problems not just at the borders
Note that
print enoise(1) has about the same odds of showing you -1 as 1 on the history, despite the different endpoint treatment, and each of -1 or 1 has about half the probability of appearing as any other multiple of 1e-6. That's not built into enoise...it's a function of print defaulting to 6 decimal digits of precision and rounding to the nearest 6 digit value. The real value returned by your expression actually has a granularity of about 1e-17, so the statistical bias of 1 vs. -1 will be about 1e-11.
Your original solution is on the right track, but you came up with the symmetric, exclusive version: 0 and 1 not included when rounding to 1e-6. If you're really trying to get a distribution which after rounding to the nearest 1e-6, could give you 0 or 1 inclusive with equal probability to any other multiple of 1e-6 then I think the answer is
random_x = enoise(0.5 + 5e-7) + 0.5
August 28, 2013 at 10:45 am - Permalink
August 28, 2013 at 12:09 pm - Permalink