
Getting wrong values from function
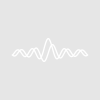
pyguy83
The code that calculates the values:
function basic(E) variable E variable z, c1 = 0.229, c2 = 2.31, c3 = 0.721, c4 = 0.0516, c5 = 126., c6 = 2.17, c7 = 0.00108, c8 = 3.33e-12, c9 = 1.62, c10 = 9.59e-8, c11 = 1.48, c12 = 299. z = c1*(E/c2)^(c3)*e^(-E/c2)+c4*e^(-(log(E)-log(c5))^2./(2.*(log(c6))^2.))+c7*log(E/c8)*(1+tanh(c9*log(E/c10)))*(1-tanh(c11*log(E/c12))) print z return z end
The code that I am running to make the new wave:
function spectra() variable i, phi_L wave phi_b, phi_inf, energy phi_L = low_energy() for (i = 0; i < 30000; i += 1) phi_b[i] = basic(energy[i]) phi_inf[i] = phi_b[i]*phi_L endfor end
For example my calculator gives a value of 0.000599384879 for the input value of 10^-8 but Igor gives inf. I have attached a file of the equation it is equation (7) on page 548. Thank you for the help!
July 21, 2015 at 01:08 pm - Permalink
July 21, 2015 at 01:28 pm - Permalink
exp(...)
instead ofe^(...)
since your variable is calledE
. Note that igor is case insensitive.July 21, 2015 at 02:16 pm - Permalink
July 23, 2015 at 06:54 am - Permalink
e^(-E/c2)
inz = c1*(E/c2)^(c3)*e^(-E/c2)
is very close to 1 for your mentioned E=1e-8,hence it will not have an effect on the final result. (I guess this is a typo and not an intended "mixing")You can do wired things with these named constants:
As an example, execute
print e
in the command window. Everything seems fine. Now copy
constant e=42
to the local procedure file (ctrl-m), compile it, and execute the command again.
Now you get "the answer".
HJ
July 23, 2015 at 07:20 am - Permalink
July 23, 2015 at 07:31 am - Permalink