
Help: Is there ways to make parallel shifts for curves
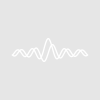
Eddie Wen
The data (waveforms, voltage vs. time) I collected from my physiological experiments not always have the same baseline. Many times, the baseline was shifted vertically due to recording noise, etc. Is there a Igor function to zero the baseline for all waveforms? Thanks.
What I typically do is that I calculate the average voltage or current of a wave before a stimulus was applied (current/voltage step, light flash, drug application, etc.). I then subtract that average pre-stimulus value from the entire trace.
Writing a function to do this for you in Igor is easy, but the function you write would depend some on how you store this kind of metadata (stimulus information), assuming you want your zeroing function to be "smart".
For now, we can probably just tell the function the time range over which we want to calculate the baseline, since if you have several waves recorded under the same conditions you probably want to use the same time range to calculate the baseline.
The other tricky thing is how do we tell the function what waves need to be zeroed? We could zero all waves in the current data folder, or zero the wave that is the basis for all traces on a given graph. Or we could pass a list of waves to the function in a string parameter and zero all of those waves. Which one of these is best depends on how you have organized your data. For my experiments, I usually zero all waves in a given data folder. Since you said you are new to Igor, if you want more background on what data folders are and how to use them, execute the following command from the Igor command line:
I've written two simple functions you can use to subtract the baseline from all numeric waves in the current data folder. Using these functions will overwrite the waves in place. If instead you want to create a copy of the data waves and zero the copy, you'll want to add in a call to the Duplicate operation within the For..EndFor loop and then pass the wave reference for the *duplicated* wave to ZeroAWave(). To use this code just copy it and paste it into an Igor procedure window. You can call it from the Igor command line like so:
This would calculate the baseline by averaging over the first second of the wave. For this to work as you expect it, you will need to have set the x scaling of your waves properly. To do that, you'll want to use the
SetScale
command. Depending on how you are acquiring your data, this might already have been done for you.Here's the code:
December 22, 2008 at 04:10 am - Permalink
December 24, 2008 at 08:22 am - Permalink