
How to check for and build/replace tables or graphs
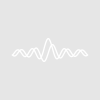
daggaz
So if I graph wave_y vs wave_x, it checks to see if its already graphed, overwrites if it is, or creates a new graph if not. Currently im getting a ton of graphs that I have to close manually..
Im aware that tables automatically update, but the question arises again if I would like a new table in case i switch to wave_a and wave_b from wave_x and wave_y.
Hope that made sense..
Note the following documentation for
Display
:This is why it's important to test for window existence first, since otherwise you'll automatically end up with windows called "dummy0", "dummy1", and so forth.
Alternatively you could loop over all graph windows (
WinList
) to see if any of these displays wave_y. But given the information you provide I would probably used named windows.This concepts translates to tables as well.
December 18, 2012 at 05:50 am - Permalink
EDIT: btw, it should be IF (V_flag ==0) if you are checking that the window does NOT exist. Took me a minute to figure out why that code wasnt working ;-)
EDIT EDIT: Ok, what do I do then if my window does exist and I want to overwrite the graphs? I cant seem to find any overwrite function in Display, is it necessary to kill the window and redraw it?
December 21, 2012 at 04:15 am - Permalink
I'm not sure what you mean by "overwrite the graphs". Some possibilities are:
December 21, 2012 at 05:41 am - Permalink
At the moment I am using dowindows/K to just kill it and then rebuilding it according to the code above, but I do wonder if this is the proper method.
December 21, 2012 at 05:43 am - Permalink
If you change the wave data the graph will automatically update. There is no need to regraph it.
Here is an example. Execute Demo() from the command line several times:
December 21, 2012 at 05:53 am - Permalink
But thanks!
December 21, 2012 at 06:58 am - Permalink
You can use TraceNameList to get the waves currently on the graph then use RemoveFromGraph to remove these traces. Finally you can add the new waves by using AppendtoGraph. Of course this isn't much different than killing the window and starting fresh.
Of course, my crude example could also be accomplished by having both waves on the graph but hiding one or the other depending on the situation.
December 21, 2012 at 08:04 am - Permalink
Good catch! I've updated my original post.
December 23, 2012 at 08:41 am - Permalink
No need to...Matlab works the way you were assuming Igor worked, and when I first started using Matlab, it took me a few tries to figure out why my graph wasn't updating when I changed the data :-). I prefer Igor's behavior as a general rule, but it's not the only way to do things.
December 23, 2012 at 10:29 am - Permalink
I am overwriting one of my waves using
integrate /d= outputwave, where outputwave is called using a string reference $outputwave_name,
and where outputwave (and its string ref) is a pre-existing wave (from the last time i used the function)
The table gets edited correctly, but the graph gets made with outputwave#1 instead of just outputwave. That screws up my trace modifications, etc, etc.
This doesn't happen with the other pre-existing waves in the same graph. Why the #(number) suffix? I tried to fix the behavior by removing and killing the wave in question beforehand, but to no avail.
EDIT: figured it out finally. I was appending the trace to the graph, and in this case the trace already existed. I had forgotten about that line when I included the dowindow logic. Moved it inside the loop and all is well.
December 25, 2012 at 08:50 am - Permalink