
How to check if a file exists
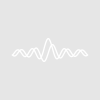
wholmgren
Maybe someone can suggest a smarter way to solve my problem: Our data folders are organized such that I have a bunch of files with names like
a1.txt, a2.txt... a122.txt, b1.txt, b2.txt, b3.txt... b235.txt, c1.txt...
I'd like to process all of the "a" files at once. Right now I have to manually enter the number of files to run my processing loop on. I'd like to automate that. I tried using IndexedFile but I couldn't figure out how to make it work for files that weren't just numbered as 001.txt, 002.txt, 003.txt...
Alternatively, is there a way to keep LoadWave from complaining with a dialog box if it doesn't find the file it's looking for?
For that, you are better off using
GetFileFolderInfo
with the /Z=1 flag. You can inspect the value of V_Flag to determine whether the file or folder was found, and the value of V_isFile if you need to be sure it's actually a file and not a folder.There are quite a few ways you could approach this. The one I thought of first was to use the following snippet. Just put it in a function somewhere and it should work (though I haven't tested it).
March 18, 2009 at 05:19 pm - Permalink
March 18, 2009 at 05:43 pm - Permalink