
How to create a blinking image marker?
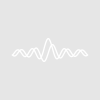
s.r.chinn
function drawdot(x,y, contrast) variable x, y, contrast variable ticks0, j variable color = 65535/2 + contrast*65535/2 // sets the blinker contrast in the autoscaled image SetDrawLayer /W=Graph2/K UserFront // clear old draw objects SetDrawLayer /W=Graph2 UserFront for(j=0;j<5;j+=1) // blink loop SetDrawEnv gstart, gname = greydot SetDrawEnv xcoord= bottom,ycoord= left,fillfgc= (color,color,color),linethick= 0.00 DrawOval /W=Graph2 x, y, x-0.02, y-0.02 // a variable greyscale fill pattern SetDrawEnv gstop DoUpdate/W=Graph2 ticks0 = ticks do while( (ticks-ticks0)<60 ) // delay one second, for example DrawAction getgroup=greydot, delete, begininsert SetDrawEnv gstart, gname = greydot SetDrawEnv xcoord= bottom,ycoord= left,fillpat=0 ,linethick= 0.00 // no fill DrawOval /W=Graph2 x, y, x-0.02, y-0.02 // effectively invisible SetDrawEnv gstop DrawAction endinsert DoUpdate/W=Graph2 ticks0 = ticks do while( (ticks-ticks0)<60 ) endfor end
Just delete the second drawing part in your for-loop and it works. Drawing and deleting is enough to let the dot blink.
Next, some suggestions:
1) You'd rather use the nifty function 'sleep' for the delay. No need for empty do-while-loops.
2) In the current state the function is extremely hard-coded to a specific case (graph named 'graph2' with bottom and left axes, also the dot size is very dependent on the axis range), but I guess you don't mind. It wouldn't be too difficult to fetch all this data for a more general approach, though (e.g. bringing the desired window to the front at start to omit all these /W flags)
3) You want the dot to blink only five times? How about forever or until something happens? You may want to read more about background functions then.
Here's my reworked function, by the way:
February 2, 2012 at 09:31 am - Permalink
Thanks for your timely and helpful response and suggestions. Your code works well. I have elected to stay with the 'ticks' timing option because the 'sleep' documentation suggests it is best suited for longer intervals rather than fractions of a second (maybe I am wrong there). I have gotten the marker display to blink at about 10Hz.
February 2, 2012 at 10:56 am - Permalink
Had you been from across the pond, we might have responded to this differently. :-)
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAHuntsville
February 2, 2012 at 12:07 pm - Permalink
February 2, 2012 at 01:17 pm - Permalink