
How to read a number from filename and output to a new wave
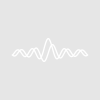
rahulsrao
I have several x and y spectral data files that were collected over time, and their timestamp is in the filename. For example, the files are named xspec1.asc, xspec3.asc, xspec4.asc and so on. The timestamps are not exactly every second but vary. Is there a way to read these filenames and extract the timestamp numbers into a separate file, say called time?
I've been looking at the various available functions - RemoveEnding will work to take out the ".asc", but I'm not sure how to extract the number, reject the "xspec", and output to a new wave. Will StringFromList or sscanf work?
Thanks
Rahul
This just extracts the first set of digits it can find (in sTest) into the result string (sNum).
HTH,
Kurt
May 17, 2015 at 12:19 pm - Permalink
In addition to KurtB's solution, you could use string indexing to extract the part of the string of interest.
Execute the following from the Igor command line read more about string indexing from the help file:
DisplayHelpTopic "String Indexing"
.After applying RemoveEnding you should have a string like xspecNNN, where NNN is the time stamp.
Any part of a string can be accessed using string indexing. For example:
print "xspec100"[5,inf]
will print "100". That is, it will print the part of the string from the 5th character to the end of the string. Indexing is 0 based -- the first character is at position "0", and "inf" is a convenient way to specify the end of the string. You could also use "strlen" to get the exact string length. Position of the final character in the string is the value reported by strlen less 1. Any contiguous part of the string can be accessed using appropriate starting and ending values inside the brackets.
Use "str2num" to convert the timestamp from a string to a numeric value.
Variable nTimeStamp = str2num( "xspec100"[5,inf] )
will assign the timestamp value ("100") to the numeric variable nTimeStamp.
Hope this helps.
May 17, 2015 at 11:45 am - Permalink
Now I need to figure out how to convert the string into a datapoint in a wave so that I can read off the filenames from all of my files and populate a wave ....
Haven't had much luck finding the solution in the help files.
Rahul
May 17, 2015 at 01:45 pm - Permalink
str2num(theString).
and set to a point in your wave such as
yourWave[index] = str2num(theString).
May 17, 2015 at 05:06 pm - Permalink
When I run it, the wave Times is created with the right number of rows, but all values are 0 instead of the number following "yspec", i.e. 1, 3, 4, etc.
Rahul
May 17, 2015 at 06:49 pm - Permalink
with this:
For easier debugging, change it to this:
Finally, I'm not sure if this use of INF is safe or not. To be safe, change it to this:
Or use sscanf, like this:
May 17, 2015 at 08:17 pm - Permalink
Thank you again! splitting up the commands does make it a lot easier to debug.
Thanks
Rahul
May 19, 2015 at 06:21 am - Permalink