
HTML table to text and numeric waves
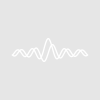
ajleenheer
Currently I'm doing the following workflow which works for one specific webpage but is pretty kludgy and probably not very general.
S_ServerResponse=ReplaceString("<td>",S_ServerResponse,";")
or S_ServerResponse=ReplaceString(" align=\"center\"",S_ServerResponse,"")
etc. etc.StringFromList(j,StringFromList(i,S_ServerResponse,"|"),";")
)Now I have a 2-D text wave with the HTML table contents. Next I'd like to convert each column to the appropriate kind of wave, either text, numeric, or date/time. Currently I'm exporting the 2D table to a CSV file using Save, then re-importing it using LoadWave such that Igor automatically determines the column type. Is there a cleaner way to do that without the save/load?
I can't think of any other automatic way to do this. I think your approach is actually pretty clever.
Is there any chance you can get the data from the web server as JSON?
October 6, 2016 at 09:30 am - Permalink
October 10, 2016 at 10:43 am - Permalink
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
October 10, 2016 at 04:30 pm - Permalink
You might get better responses if you include a link to the source HTML that you're processing, and if you clarified exactly what it is that you want the output to look like. Reading only your code, it's hard to get an idea of what the source data looks like.
October 11, 2016 at 05:59 am - Permalink
I've attached an example HTML file similar to what I'm processing that contains some nonsense data. For output, I want each column loaded into Igor as the appropriate kind of 1D wave. My code works relatively well on this example, other than the column with values separated by commas. I've only tested it on a couple other webpages. To load the example file, run from the command line:
October 11, 2016 at 10:48 am - Permalink
October 11, 2016 at 12:03 pm - Permalink
October 11, 2016 at 02:39 pm - Permalink