
Import replicate samples into separate Data Folders
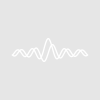
quoderatmac
I'm currently using this block of code I found on this forum. This allows me to collect data on one replicate in one Excel spreadsheet, and then import all of these spreadsheets into Igor as separate Data Folders. If I could get this code to parse the three replicates in one spreadsheet, I could cut down my experimental time and still use the same "downstream" Igor code I wrote to process data in individual Data Folders.
// GetLeafName(pathStr) // Utility routine that returns the "leaf" of a path. // For example, if the path is "hd:Folder A:Folder B:File A", this returns "File A". // The path separator is assumed to be colon. // If no leaf name is found, returns "". // It is assumed that the input path does NOT end with a colon. Function/S GetLeafName(pathStr) String pathStr String leafName Variable pos leafName = "" pos = strlen(pathStr) - 1 // Search from the end do if (CmpStr(pathStr[pos], ":") == 0) // Found the colon? leafName = pathStr[pos+1, strlen(pathStr)] break // Break out of loop. endif pos -= 1 while(pos > 0) return leafName End // LoadDataSetFromFile(pathName, fileName) // This function loads the waves from a sample data file into a new IGOR data folder. // It creates the data folder, giving it the name of the file. // It then loads the data from the file into the new data folder. // // If pathName is "" or fileName is "", it will put up a dialog from which you can // choose the file to be loaded. // If both pathName and fileName are not "", then it will load the file without // putting up a dialog. In this case, pathName must contain the name of an IGOR // symbolic path that you have created which points to the folder containing the // sample data. fileName must be the name of a file within that folder. Function LoadDataSetFromFile(pathName, fileName) String pathName // Name of an existing IGOR symbolic path or "" String fileName // Name of file within folder pointed to by symbolic path or "" // If either of the input parameters is "", put up a dialog to get file. if ((strlen(pathName)==0) %| (strlen(pathName)==0)) Variable dummyRefNum String pathToFolder Open/R/D/M="Choose data file" dummyRefNum // This sets an automatically created local variable named S_fileName. if (strlen(S_fileName) == 0) // User cancelled? return -1 endif // Now break the full path to file down into path to folder plus file name. // This is done by searching for the last colon in the full path. fileName = GetLeafName(S_fileName) if (strlen(fileName) == 0) Print "LoadDataSetFromFile bug" return -1 endif pathToFolder = S_fileName[0, strlen(S_fileName) - strlen(fileName) - 1] // Make sure there is an IGOR symbolic path pointing to the folder NewPath/O/Q CurrentDataFilePath pathToFolder pathName = "CurrentDataFilePath" endif String extension = "." + ParseFilePath(4, fileName, ":", 0, 0) // e.g., ".dat" // Save the current data folder so we can restore it below. String savedDataFolder = GetDataFolder(1) // If it does not already exist, make a data folder to contain the waves from the file. // The new data folder is created in the current data folder with the name the // same as the name of the file we are about to load. // The /S flag sets new data folder as the current data folder. String dfName = RemoveEnding(fileName, extension) NewDataFolder/O/S :$dfName // This loads data set from the file into the current IGOR data folder // which is the data folder we just created above. The wave names come // from the first line of the data in the file because of the /W flag. //########################################## XLLoadWave/Q/S="Data"/R=(A20,BH1050)/W=19/O/D/P=$pathName fileName // Example from experimental data //########################################## // Restore the original data folder. SetDataFolder savedDataFolder return 0 End // LoadDataSetsFromFolder(pathName) // Loads data from all of the files in the folder associated with the specified symbolic path. // pathName is the name of an IGOR symbolic path which you can create with the New Path // dialog in the Misc menu. // If pathName is "", it puts up a dialog from which you can choose the folder. // // NOTE: This function assumes that ALL of the files in the specified folder are data // files and thus loads them all. Function LoadDataSetsFromFolder(pathName) String pathName // Name of an existing IGOR symbolic path or "" // If pathName is "", allow user to create a new symbolic path to the folder containing the runs of data. if (strlen(pathName) == 0) NewPath/O/Q/M="Choose folder containing data files" CurrentDataFilePath PathInfo CurrentDataFilePath // Check to see if user created the path. if (V_flag == 0) return -1 // User cancelled. endif pathName = "CurrentDataFilePath" endif Variable i, numFilesLoaded String fileName //########################################## String extension = ".xls" // You need to change this for your data. //########################################## numFilesLoaded = 0 i = 0 do fileName = IndexedFile($pathName, i, extension) if (strlen(fileName) == 0) break endif LoadDataSetFromFile(pathName, fileName) i += 1 numFilesLoaded += 1 while(1) return numFilesLoaded End <pre><code class="language-igor">
If you need further assistance, you are likely to get better help if you include an example Excel file that can be used with the code you've already posted and a description of exactly what you are looking to do as far as the output in Igor is concerned.
October 31, 2016 at 10:37 am - Permalink