
Importing xy waves stored in a lot of files
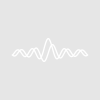
warakurna
I'm a freshman to Igor and currently I'm working on a procedure to import IR data (wavenumber vs. absorbance) from simple delimited text files. The files include one spectra with two columns (wavenumber and absorbance). The file ending is ".dpt". Setting up a method to load spectra out of one file succeeded:
<br /> // ------ IR spectra XY data loader for one spec -----<br /> <br /> Function Load_IR(genericname, path)<br /> String genericname // Name of file to load or "" to get dialog<br /> String path // Name of path or "" to get dialog<br /> <br /> String file=genericname+".dpt"<br /> <br /> Wave Wavenumber, Absorbance<br /> String wnstr="_cm-1"<br /> String Wavenumberwave=genericname+wnstr<br /> String Abstr="_A"<br /> String Absorbancewave=genericname+Abstr<br /> <br /> // Load the waves and set the globals<br /> LoadWave/J/D/O/P=$path/B="C=1, N=Wavenumber; C=1, N=Absorbance;" file<br /> duplicate/o Wavenumber $Wavenumberwave<br /> duplicate/o Absorbance $Absorbancewave<br /> <br /> End<br />
I even got a method which plots the imported data:
<br /> // ------ IR spectra XY data loader & grapher for one spec -----<br /> <br /> Function LoadAndGraph_IR(genericname, path)<br /> String genericname // Name of file to load or "" to get dialog<br /> String path // Name of path or "" to get dialog<br /> <br /> String file=genericname+".dpt"<br /> <br /> Wave Wavenumber, Absorbance<br /> String wnstr="_cm-1"<br /> String Wavenumberwave=genericname+wnstr<br /> String Abstr="_A"<br /> String Absorbancewave=genericname+Abstr<br /> <br /> // Load the waves and set the globals<br /> LoadWave/J/D/O/P=$path/B="C=1, N=Wavenumber; C=1, N=Absorbance;" file<br /> duplicate/o Wavenumber $Wavenumberwave<br /> duplicate/o Absorbance $Absorbancewave<br /> <br /> // Begin Graphing<br /> Display/k=1/w=(50,20,450,420) $Absorbancewave vs $wavenumberwave;<br /> // SetAxis for MIR<br /> SetAxis left -0.2,3;DelayUpdate<br /> SetAxis bottom 4000,1000<br /> // SetAxisLabels<br /> Label left "Absorbance";DelayUpdate<br /> Label bottom "Wavernumber (cm\\S-1\\M)";DelayUpdate<br /> // Annotate graph<br /> Legend/C/N=text0/A=MC<br /> Textbox/A=LT "Loaded from " + S_fileName<br /> return 0 // Signifies success.<br /> End<br />
But I fail to set it up for all files (with this ending) in one folder. I already tried to adopt code snippets but without success.
Does somebody know how to solve this problem...
Thanks for comments...
Can you clarify what you mean when you say you "fail to set it up for all files"? What goes wrong? Which of your two functions doesn't provide the correct results? Also it would be helpful if you provided a small file that contains data in the format you are loading so that others can use your code directly to test it.
By the way, when you are typing in Igor Pro code on this web site, it's best to enclose the code in <igor></igor> tags, so that it will be colored correctly for Igor. You used <ccode></ccode> tags, which color keywords and functions common to Igor and C, but miss a lot of Igor specific keywords, etc.
July 3, 2009 at 06:14 am - Permalink
FWIW, the packages SpXZeigR and/or LinkVS and/or Scroll Traces may be of interest to you. They have been designed from a basis of using Igor to work on data from chemical spectroscopies.
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
July 3, 2009 at 06:38 am - Permalink
Sorry, I did not see the igor tag.
The two functions I posted work good. But I'm not able to figure out how to design a function which loads all the files in one folder or path.
A file with the data is attached.
July 3, 2009 at 06:51 am - Permalink
From that code snippet, you want to replace
with a call to your two functions. Also, you can probably comment out the following line if you don't need the directory name printed to the command line:
I would suggest that you change your two functions to take a fileName parameter, instead of genericname. Then to get everything started you would execute something like the following at the Igor command line:
This assumes that you do not want to recurse into subdirectories. You may want to change the name of the function as well and if you do so then obviously you need to change what you type into the command line.
Let me know if I've misunderstood the problem you're having.
July 3, 2009 at 09:25 am - Permalink
You didn't misunderstand! That's exactly what I want to do. The Snippet you linked is a good start. I just inserted it in my procedure file as is and the "PrintFoldersAndFiles" function works good. I get a list of all dpt type files in my history.
But, how to replace the printf term you mentioned. It does not work with simply replacing this line
with
from the function in the first post.
It seems to be a bit more complex. Do you have a hint or an explanation.
By now, my knowledge on writing and/or modidying procedures is very limited! Sorry for that...
July 6, 2009 at 02:04 am - Permalink
July 6, 2009 at 09:49 am - Permalink