
Loading am/pm time columns into a matrix
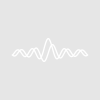
LaMP
The first few columns are dedicated to time: "day, month, year, hour, minute, second, Am/pm" and the rest contain data: data1, data2, data3...etc
When I load the text file, the column with the am/pm information is left blank. I presume this is because I am only telling the matrix to load numerical data.
Is there a way to load this information (am/pm) into the matrix, or even better, convert the time stamp to a 24hr format..
I copied in my code below, and I attached an example text file
Function Load_PAM_File(filename, refnum) // load single PAM file
String filename
Variable refnum
print "Loading: " + filename
loadwave/v={"/: \t", "", 1,1}/q/a/n=Temp_PAMDataMatrix/m/o/j/k=1/l={0,0,0,0,0} filename // loads PAM data as a matrix
End
Thank you in advance
The use of AM/PM is unfortunate. To handle this requires loading the AM/PM column as a text wave and then using it to adjust the date/time wave.
I wrote a file loader (see below) for your file. It loads the date/time into a 1D wave and the rest of the data into a matrix.
It calls LoadWave three times:
1. Load the date/time data
2. Load the AM/PM column
3. Load the remaining columns as a matrix
April 5, 2016 at 03:58 pm - Permalink
Thank you for your quick reply, and for the time you took to write the code. However, I am having a bit of trouble to implement the code or at least getting it to work!
I have attached the igor procedure, with your code copied into it.
Thanks very much
April 6, 2016 at 12:32 am - Permalink
It's always helpful if you specify exactly what the problem is, for example, what error message you or what incorrect behavior you are getting.
I can't compile your file because it uses functions (e.g., Browse4Folder) that I don't have.
Anyhow, your PAM_loader.ipf file does not even call the code that I wrote.
Start by copying the code I wrote to a separate procedure file in a new experiment. Compile that procedure file and choose Data->Load Waves->Load PAM File. Select a file and load the data to confirm that it works correctly. Note what output waves are created.
Next open the separate procedure file and carefully read the code so you understand what the inputs are and what the outputs are. If necessary, step through the LoadPAMFile using the debugger to understand it.
Then try to integrate my code into your code. If this fails, create a simplified, self-contained example that I can run and debug and post it with a specific question.
April 6, 2016 at 10:34 am - Permalink
Thank you for your reply.
Apologies for not being specific about the errors.
I have managed to get the code to work. I am now trying to implement your code into my loop function so that it would load all files in the folder into a single time wave.
In my loop function, it looks for a folder containing the data. However, when I try to combine the loop function with your code it keeps looking for individual files and does not load all files in the folder.
I also get a wavewrite error "index out of range for wave "dateandtime".
I call the function using "loaddateandtime()"
The procedure file is attached PAM_loaderV2.ipf
I am a bit stuck and would be very grateful for a bit of advice.
Thanks again
April 7, 2016 at 12:34 pm - Permalink
I recommend that you read the code and comments. It is most useful to read the higher level functions first which means starting at the bottom of the file.
April 7, 2016 at 11:20 pm - Permalink
Thank you very much for all this work. The function works perfectly.
April 8, 2016 at 01:23 am - Permalink