
LoadWave error - how to determine number of lines in a file before opening?
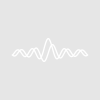
jdorsey
if(WaveExists(SPP200Paths)) sort /A SPP200Paths, SPP200Paths tmpWaveList = "" for(i =0;i<dimsize(SPP200Paths,0);i+=1) wfprintf currentFile, "%s" /R=(i,i) SPP200Paths LoadWave/J/M/U={0,0,1,0}/D/A=SPP200tmp/E=0/K=0/V={"\t,"," $",0,1}/L={19,20,0,0,0}/Q currentFile tmpWaveList += "SPP200tmp" + num2str(i) + ";" endfor Concatenate /NP=0 /KILL tmpWaveList, SPP200 SetScale /P y, 0,1,SPP200 ModifyControl CAPS_Analyse_CAS disable=0 // columnLabel = "SPP200_Ch1 [counts]" // display SPP200[][(FindDimLabel(SPP200,1,columnLabel))] endif
The file paths contained in the text wave SPP200Paths can refer to files which contain header data, but nothing else. I don't want to read the header data in this part of the function, - that's taken care of elsewhere. When the loop hits a file containing nothing but header information, I get the error "LoadWave error: "no data was found in file"". If I try to get rid of this warning using the /C flag to LoadWave, the Concatenate command generates errors (Concatenate error: "expected wave name").
So at last to the question - is there an elegant way to determine the number of rows in these delimited text files before calling LoadWave? If I could put some sort of IF statement in the loop it'd fix the problem i.e.
if (LinesInCurrentFile > 20) Loadwave ... endif
But how would one go about determining LinesInCurrentFile?
If that is not good enough, you will have to parse the file yourself. Here is an example of a function that looks for a line that starts with two numbers:
This is called like this:
October 5, 2010 at 08:16 am - Permalink
October 5, 2010 at 09:46 am - Permalink
You should not use /C. It is intended for experiment recreation procedures only.
Omit /C and use GetRTError.
October 5, 2010 at 12:59 pm - Permalink
Thanks for your help. I got so annoyed by the debugger popping up during expected occurrences (empty files) that I went with your other suggestion.
October 7, 2010 at 08:04 am - Permalink
GetFileFolderInfo
and check the output variable V_logEOF to see if it's above some threshold.October 8, 2010 at 06:43 am - Permalink