
Loop in a Wave
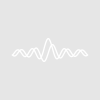
igorman
I'm new to Igor. For my project I need to create a loop that goes through every point in a wave and compares every value of the wave to a threshold. My output should be how many data points of that wave go over that threshold.
I have about 5.9x10^6 data points that define the trace (graph). There are two threshold values that I wish to use and see at what points/the wave goes above/below that and at what place it does (so I would need an index for this.) For now I"m hoping to just work on the above part.
Simple Example:
---------------
Wave1
1
2
3
4
5
6
7
8
9
output = 2 // because 8 and 9 are over the threshold that is 7.
--------------
So far, this is what I have as my code My issue is that the code stops immediately after it seeks the first point where the threshold is exceeded. Although I have been able to accommodate an index to show where the threshold value is met I've not been able to define the # of times this happens. I would be grateful for any tips. I'm new to the Igor environment, and still getting used to the way of things.
Function GetPoint(inwave, threshval) Wave inwave Variable threshval Variable point=0 Variable maxindex = numpnts(inwave)-1 print maxindex if (inwave[0]==threshval) return 0 Endif do point+=1 if(point>maxindex) return -1 Endif while(inwave[point]<threshval) return point End ----------------------------
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAHuntsville
May 21, 2013 at 10:43 am - Permalink
MatrixOP/O aa=sum(greater(inWave,trs))
Print aa[0]
I hope this helps,
A.G.
WaveMetrics, Inc.
May 21, 2013 at 04:54 pm - Permalink
Thanks for all the opinions. I've tried them out, but I'm still having problems. I posted my new questions on a new forum topic, but here it is again (for those who have followed my problem so far):
I'm new to the Igor environment. I'm currently having a project where I have a wave of data points, about 5.9 x 10^6 points. I had to write a program that finds the places at which a particular value(s) was crossed, for example something like 557.007, 580.444. I have to find the points where these values are crossed both as the numbers increase or on the way of decrease (similar to going up a curve, or going down a curve). I was advised to use the FindLevels operation to do this and all seemed be going well. But when my output was seen on the table, I could only get the iteration to go up to 127 points, while my data wave consists of 5.6 x 10^9 points. Is there a particular limit to the Findlevels operation? I was thinking of using a for loop + findlevels mechanism to accommodate for this, but am not too sure how to get it done. I would be grateful for tips and explanations.
The data looks like this:
0 562.21
1 558.002
2 555.361
3 551.643
4 555.463
5 562.009
6 556.385
7 556.553
8 552.292
9 552.115
10 557.492
11 556.408
12 556.867
13 557.39
14 557.722
15 557.043
16 564.752
...
5.9E6 557.689
Here is my code:
May 27, 2013 at 11:58 am - Permalink
The wave should have been created by FindLevels and so does not need to be made again.
Since you have specified no number of points Make defaults to 128 point. This truncates whatever FindLevels produced.
May 27, 2013 at 12:22 pm - Permalink
-------
Yes I can understand what you say. the reason I thought I did Make/O LevelCrossings1a, was to see the distributions of different waves, as for each findlevels statement like in LevelCrossings1a, I'm looking for that particular point while traversing the curve in the up direction, while in the second LevelCrossings1b it is for the down direction. I wanted to see it as separate waves so I can distinguish the different points. Does this make it complicated?
May 27, 2013 at 12:46 pm - Permalink
May 27, 2013 at 01:08 pm - Permalink