
Matrix problem
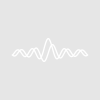
jgladh
Hi,
I have a small problem that I don't know how to solve. I want to do some matrix calculations, where i have units and waves in the same matrix, e.g. in a 2x2 matrix, position [0][0]=1, [0][1]=sin(x), [1][0]=sin(x), and [1][1]=1. Is this possible to do?
Grateful for any sugestion
Jörgen
I am not sure I know what you mean by "units and waves in the same matrix". From the example you provide it seems that you have constants (i.e., 1) and computed values [sin(x)] in the 2x2 matrix.
If your sin(x) is indeed a wave then you may want to use a 3D wave, e.g., of dimensions (2,2,N) where each layer of the wave would correspond to a particular x value.
This gives you a 3D wave where each layer will be a 2x2 matrix as you described above.
I hope this gives you one idea for implementation. Let us know if you need something different.
AG
December 13, 2019 at 10:59 am - Permalink
Hi AG,
Thank you for this suggestion, but I'm not sure if I been clear enough, or I don't grasp the concept. I want to have matrices with just numbers, like 0 or 1 at some positions and a function in others. In the end I want to do matrix calculations.
I attached a figure and hope that its make a clear picture of what I want to do.
December 16, 2019 at 06:39 pm - Permalink
You want Igor to be Excel, and it isn't.
Instead, write a function that fills in your matrix with the needed info, something like
That assumes that the layers scaling was already set before calling the function. You could add the SetScale command to this function if you want.
December 17, 2019 at 09:36 am - Permalink
In reply to You want Igor to be Excel,… by johnweeks
Hi johnweeks,
thank for the input, but no I don't want it to be Excel, I actually converting an Python script into my IGOR experiment. In Python you can do 2x2 matrices and set the every position in the matrix with a variable. Then the variable can be either an constant or an function. But again thanks a lot for your input, much appreciated.
Best
Jörgen
December 17, 2019 at 11:37 am - Permalink
In reply to Hi AG, Thank you for this… by jgladh
Just curious, what is the x in the expression above? Is that covered by wave scaling? A.G.'s and John's examples above assume that.
December 17, 2019 at 11:38 am - Permalink
Hello Jörgen,
Numeric waves in Igor contain numbers, not functions. As such you can have the assignments as shown above where sin() is evaluated once at runtime for the parameter that you pass to it. In other words,
ddd[1][0][]=sin(z)
is evaluated once for each z-value in the range (subject to wave scaling and all) at the time you execute this instruction.
If you are trying to translate from Python a concept that does not exist in Igor, you could probably create an inefficient translation using a text wave, e.g.,
and then when you want to use this "creature" you would have to convert the text into numeric or command. Here is a simple 1D example:
This is clearly a kluge, but it might help with your code translation.
A bit more elegant solution would be to create a dependency. You can find out more about that by executing:
DisplayHelpTopic "Dependencies"
AG
December 17, 2019 at 12:05 pm - Permalink
In reply to Hello Jörgen, Numeric waves… by Igor
Hi AG,
Thanks a lot, much appreciated
Best
Jörgen
December 17, 2019 at 01:25 pm - Permalink
In reply to jgladh wrote: ... [0][0… by ChrLie
Hi ChrLie,
the x is just a variable e.g. in the range 0 to 90 degrees.
Best
Jörgen
December 18, 2019 at 01:11 pm - Permalink