
Minimization problem: Match parts of two waves via scale and offset
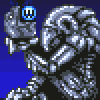
chozo
I want to find the offset and scale values for the first wave to match programmatically, i.e., a function which calculates these values from the two waves (and optionally how much of the outer values can be used in the calculation).
My ideas are to use a temporary wave to calculate the difference and use some complicated iteration until the difference is very small or abuse functions like 'Optimize' in some way (maybe I’m just too tired today, but I can't find a way ;). Since noise is included, there has to be some criteria for this. Also there may be cases where there is no exact match possible, which also needs to be considered. In Excel there is the solver add-in, where the program fiddles with some values until a minimum/maximum etc. is achieved for some result value. Basically I need this for the sum of the difference for these two waves (in parts). I hope someone can point me to an elegant solution for this.
Optimize
looks good to me.A first rough sketch with
results in following graph.
It is not completely automatized yet as you still need to find pSkipStart and pSkipEnd manually.
September 3, 2014 at 10:03 am - Permalink
Optimize
before. It is no problem that you have to give the omitted range manually. I guess there is no way to find this automatically for a wide range of data.I have modified the code a bit, and giving Optimize a rough parameter size using the /R flag gives a great result. Now I only need to find an elegant way to sneak two arbitrary named waves into the worker function.
September 3, 2014 at 07:42 pm - Permalink
FindAPeak
and assume some width.For the sneak in part, unfortunately
Optimize
does not accept wave reference waves as parameter wave.So we have to resort to wave notes:
September 4, 2014 at 02:49 am - Permalink
I have fitted one wave to the other using an offset and scale, then excluded the points that are most extreme (positive peak). Then repeated the fit and exclusion a few times.
Example code as follows - I hope it is self explanatory:
Hope this makes sense.
I tried to get this to work using a mask wave, but failed, hence my odd use of 'wave1iter'.
Regards,
Kurt
September 4, 2014 at 07:13 am - Permalink
September 4, 2014 at 06:14 pm - Permalink
A better solution (at least for this shape of data) is to exclude the most extreme points irrespective of sign.
One could also extend this algorithm to auto stop excluding points when, for example, the largest outlier is 2 x StDev of the residual (say) - this removes another 'human' parameter from the algorithm. (Perhaps this factor should be scaled by number of data points - but my head starts to hurt when I start thinking about that).
New example code as follows:
Apologies for the pint-point typo... perhaps my fingers were hinting at where I should go after work ;)
Regards,
Kurt
September 5, 2014 at 12:33 am - Permalink
September 8, 2014 at 02:04 am - Permalink