
Multi-Experiment Process, ask if I want to save change to experiment "Untitled" all the time.
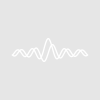
chrisNell
I have done a batch function based on the explanation to sequentially open experiment file find in: Choose File->Example Experiments->Programming->Multi-Experiment Process.
I manage to make a nice code using this example. Everything works well when I follow the tutorial but when I want to implement my own function I have a problem. It comes from a Hook function in my experiment:
static Function IgorStartOrNewHook(igorApplicationNameStr)
String igorApplicationNameStr
...
End
If I use this function, igor will ask before every new experiment:
Do you want to save change to experiment "Untitled"?
somebody have any idea why? Thanks again
Chris
Your function may be setting globals you don't expect (like V_Flag).
You can use the ExperimentModified operation to tell Igor you don't consider the current experiment in need of saving.
--Jim Prouty
Software Engineer, WaveMetrics, Inc.
August 14, 2015 at 04:14 pm - Permalink
Hello Jim,
Thank you for your reply, I already try ExperimentModified without success but maybe I called it at the wrong point, so I will try other combinations. The whole function is bellow, it is the function taken from Igor: Choose File->Example Experiments->Programming->Multi-Experiment Process. The modification is only a simple callback. It mimics the real modification that I have in my code but it may be easier for you to understand this code provided directly by Igor developers.
August 17, 2015 at 12:51 am - Permalink
August 18, 2015 at 01:02 am - Permalink
I have solved my problem by adding
"ExperimentModified 0"
at the end of the hook function that was responsible of my problem! Thanks a lot for your reply, best
Christophe
August 18, 2015 at 01:33 am - Permalink
<pre><code class="language-igor"></code></pre>
tags to your code posting so that we can read it. Without those tags, certain characters are interpreted as formatting instead of code. See http://www.igorexchange.com/node/25 for more info.John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
August 18, 2015 at 09:20 am - Permalink