
Mysterious issue with loading a list of files (resolved: changing name of wave doesn't change the references to it)
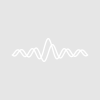
Jay
Fri, 09/08/2023 - 09:42 pm
I made a function that takes a string and then loads all the files which have filenames starting with that string. It works, EXCEPT for the very last file. The puzzling part is that every matching filename shows up in the list, matchingWavesList. I don't understand why the last filename in this list fails to load.
// load_allWavesLikeTemplate:
// Say you have a bunch of text-delimited files that all start with "file_".
// This function loads all of those files, and makes a reference wave that
// points to all of them.
// parameters:
// nameTemplate : a string that tells how all the file names should start
// pathSTring : a string w/ the complete path the folder with all the files
// df : a data folder in igor where all the loaded waves will be kept
// outputs:
// - one wave for each file name that matched the template
// - *nameTemplate*_All : a wave of references that points to all the loaded waves
Function load_allWavesLikeTemplate(nameTemplate, mode, [pathString, df, wOutName])
String nameTemplate, mode, pathString, wOutName
DFREF df
DFREF saveDFR = GetDataFolderDFR()
if (!ParamIsDefault(pathString))
NewPath/O myPath, pathString
else
NewPath/O myPath
endif
if (!ParamIsDefault(df))
SetDataFolder df
endif
String allFileList = IndexedFile(myPath, -1, "????")
String/G matchingWavesList = "" // #todo: remove this /G later
String fileName
Variable i, imax = ItemsInList(allFileList), isMatch
for (i=0; i<imax; i+=1)
fileName = StringFromList(i, allFileList)
isMatch = StringMatch(fileName, nameTemplate + "*")
if (isMatch)
matchingWavesList = AddListItem(fileName, matchingWavesList)
endif
endfor
// Put the file lists in order
matchingWavesList = SortList(matchingWavesList, ";", 16)
// Make a waves to store references to all the loaded waves
String refWaveName
if (!ParamIsDefault(wOutName))
refWaveName = wOutName + "_All"
else
refWaveName = nameTemplate + "_All"
endif
Make/WAVE/O/N=(itemsInList(matchingWavesList)) $refWaveName
Wave/WAVE refWave = $refWaveName
// Load all the waves
imax = ItemsInList(matchingWavesList)
String wName
for (i=0; i<imax; i+=1)
fileName = StringFromList(i, matchingWavesList)
// Print "fileName = " + fileName // #todo: remove this print statement later
LoadWave/Q/J/M/D/N=wIn/K=0/P=myPath fileName
Wave wIn0
if (!ParamIsDefault(wOutName))
wName = wOutName + "_" + num2str(i)
else
wName = fileName // I may decide to use a different name
endif
if (StringMatch(mode, "1d"))
Make/O/D/N=(DimSize(wIn0,1)) $wName = wIn0[0][p]
elseif (StringMatch(mode, "2d"))
Rename wIn0, $wName
endif
Wave oneWave = $wName
refWave[i] = oneWave
endfor
KillWaves wIn0
End
// Say you have a bunch of text-delimited files that all start with "file_".
// This function loads all of those files, and makes a reference wave that
// points to all of them.
// parameters:
// nameTemplate : a string that tells how all the file names should start
// pathSTring : a string w/ the complete path the folder with all the files
// df : a data folder in igor where all the loaded waves will be kept
// outputs:
// - one wave for each file name that matched the template
// - *nameTemplate*_All : a wave of references that points to all the loaded waves
Function load_allWavesLikeTemplate(nameTemplate, mode, [pathString, df, wOutName])
String nameTemplate, mode, pathString, wOutName
DFREF df
DFREF saveDFR = GetDataFolderDFR()
if (!ParamIsDefault(pathString))
NewPath/O myPath, pathString
else
NewPath/O myPath
endif
if (!ParamIsDefault(df))
SetDataFolder df
endif
String allFileList = IndexedFile(myPath, -1, "????")
String/G matchingWavesList = "" // #todo: remove this /G later
String fileName
Variable i, imax = ItemsInList(allFileList), isMatch
for (i=0; i<imax; i+=1)
fileName = StringFromList(i, allFileList)
isMatch = StringMatch(fileName, nameTemplate + "*")
if (isMatch)
matchingWavesList = AddListItem(fileName, matchingWavesList)
endif
endfor
// Put the file lists in order
matchingWavesList = SortList(matchingWavesList, ";", 16)
// Make a waves to store references to all the loaded waves
String refWaveName
if (!ParamIsDefault(wOutName))
refWaveName = wOutName + "_All"
else
refWaveName = nameTemplate + "_All"
endif
Make/WAVE/O/N=(itemsInList(matchingWavesList)) $refWaveName
Wave/WAVE refWave = $refWaveName
// Load all the waves
imax = ItemsInList(matchingWavesList)
String wName
for (i=0; i<imax; i+=1)
fileName = StringFromList(i, matchingWavesList)
// Print "fileName = " + fileName // #todo: remove this print statement later
LoadWave/Q/J/M/D/N=wIn/K=0/P=myPath fileName
Wave wIn0
if (!ParamIsDefault(wOutName))
wName = wOutName + "_" + num2str(i)
else
wName = fileName // I may decide to use a different name
endif
if (StringMatch(mode, "1d"))
Make/O/D/N=(DimSize(wIn0,1)) $wName = wIn0[0][p]
elseif (StringMatch(mode, "2d"))
Rename wIn0, $wName
endif
Wave oneWave = $wName
refWave[i] = oneWave
endfor
KillWaves wIn0
End
If mode=="2d" then the KillWaves statement at the end kills the last wave loaded. The fact that you have renamed the wave does not change the fact that the wIn0 wave reference refers to that wave. You could fix this by changing:
Rename wIn0, $wName
to
Duplicate/O wIn0, $wName
If that does not solve the problem then I recommend you single-step through the second loop using the debugger:
DisplayHelpTopic "The Debugger"
September 9, 2023 at 06:30 am - Permalink
Thank you hrodstein! That was exactly the issue.
I've never used, or even heard of, the debugger. I look forward to learning about it, and will try it out the next time I have a mysterious issue.
September 10, 2023 at 10:27 am - Permalink