
Name of wave and wave scalling
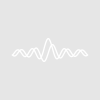
Sergey
I'm beginner in IGOR and working on analysing of data of photoemission spectra. I have a lot of files and I am making merging all of these files into one 2D matrix. There are information about this spectrum (for example name of transition, excitation energy) and two columns in source files: energy (axis X) and intensity (axis Y).
There are two main problem: automatically title wave by text from source file(I don't know how I can read string variable from source file);
set scale (X) by first column from source file(As I know it's impossible with function SetScale)
Does anybody know solving for these problems?
Thanks in advance!
If you have not already done it, do the Igor Pro Guided Tour. It is indispensible. Choose Help->Getting Started.
Most likely you will have to read the title using FReadLine. For an example see http://www.igorexchange.com/node/908.
If I understand correctly you would want to load the first column into a numeric wave. Then:
June 20, 2013 at 11:44 am - Permalink
And once more question about title. Just Iwant to load strint from first line of this file (see attached). Only "In 4d", without "# Transition ". And use this text like title of new wave.
I use this code:
June 20, 2013 at 01:33 pm - Permalink
Looking at your text file. You can break up the problem into two parts:
1. Reading the important header info
2. Readings the data in.
For your question about the the header info you want one way to do this is to treat the header portion as an import as text wave and you can read in that portion. Once you have that in you can manipulate the text string to what you wan to extract. If for example the formatting is always the same then you can trim the text the needed number of characters from the beginning. Take off the first 35 characters and you are left with In 3d5/2.
Then run a second load wave function that pulls in only the data.
While maybe not the most compact and elegant approach I have found it useful in my learning to break apart the problem and emulate what the point and click process does to help me learn.
June 20, 2013 at 04:08 pm - Permalink
June 20, 2013 at 08:31 pm - Permalink
June 23, 2013 at 06:48 am - Permalink