
NIDAQ triggered analog data aquisition using FIFO
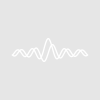
JR
I'm using Igor to run an automated behaviour setup for mice which means driving and scanning different hardware modules using IGOR and National Instruments equipment (PCI card PCI-6229 and DAQ board BNC2090A).
With this I've run into a problem setting up a triggered scan of analog channels: as the animals determine the length of one trial ideally we'd like the scanning to be of variable length (depending on the trigger). So far I only succeeded in setting up a scan using WAVES but these scans are always the same length (i.e. the length of the wave).
The code I used for this is as follows:
FUNCTION InitHardware() fDAQmx_ResetDevice("dev1") END FUNCTION TrialData() NVAR EventCount = root:EventCount Wave TmpData, AI0_Tmp, AI1_Tmp, AI2_Tmp, AI3_Tmp, AI4_Tmp, AI5_Tmp, AI6_Tmp, AI7_Tmp, AI8_Tmp, AI9_Tmp, AI10_Tmp, AI11_Tmp, AI12_Tmp, AI13_Tmp, AI14_Tmp, AI15_Tmp DAQmx_Scan /DEV="dev1" /TRIG={"/Dev1/PFI0"} WAVES="AI0_Tmp, 0/RSE; AI1_Tmp, 1/RSE; AI2_Tmp, 2/RSE; AI3_Tmp, 3/RSE; AI4_Tmp, 4/RSE; AI5_Tmp, 5/RSE; AI6_Tmp, 6/RSE; AI7_Tmp, 7/RSE; AI8_Tmp, 8/RSE; AI9_Tmp, 9/RSE; AI10_Tmp, 10/RSE; AI11_Tmp, 11/RSE; AI12_Tmp, 12/RSE; AI13_Tmp, 13/RSE; AI14_Tmp, 14/RSE; AI15_Tmp, 15/RSE" Duplicate/O AI0_tmp, Seal Seal=(AI0_Tmp<2.5)||(AI1_Tmp<2.5) //does quick online analysis to determine the point when BOTH beams have re-sealed -> equation equals 0 if both beams give more than 2,5V (i.e. have resealed) concatenate/O/DL {AI0_Tmp, AI1_Tmp, AI2_Tmp, AI3_Tmp, AI4_Tmp, AI5_Tmp, AI6_Tmp, AI7_Tmp, AI8_Tmp, AI9_Tmp, AI10_Tmp, AI11_Tmp, AI12_Tmp, AI13_Tmp, AI14_Tmp, AI15_Tmp}, TmpData //adds all waves together in one large wave to make offline analysis easier Save /O/C/P=SaveDataPath TmpData as "trial_"+num2str(EventCount)+"_Trialdata.ibw" Save /O/C/P=SaveDataPath Seal as "trial_"+num2str(EventCount)+"_BeamSeal.ibw" //saves all waves EventCount+=1 return 0 END FUNCTION StartDataRec() InitHardware() variable/G EventCount EventCount=0 //creates the counting variable needed to sort the waves according to the trial number NewPath/O/M="Pick folder to save data" SaveDataPath Make /O /N=3000 AI0_Tmp, AI1_Tmp, AI2_Tmp, AI3_Tmp, AI4_Tmp, AI5_Tmp, AI6_Tmp, AI7_Tmp, AI8_Tmp, AI9_Tmp, AI10_Tmp, AI11_Tmp, AI12_Tmp, AI13_Tmp, AI14_Tmp, AI15_Tmp, TmpData SetScale /P x, 0,0.001, "s", AI0_Tmp, AI1_Tmp, AI2_Tmp, AI3_Tmp, AI4_Tmp, AI5_Tmp, AI6_Tmp, AI7_Tmp, AI8_Tmp, AI9_Tmp, AI10_Tmp, AI11_Tmp, AI12_Tmp, AI13_Tmp, AI14_Tmp, AI15_Tmp, TmpData SetBackground TrialData() ctrlbackground period=20,stop ctrlbackground period=0,start END
In short, I start it one by calling StartDataRec() and then it keeps running the scan in TrialData() every time a trigger comes in over the PFI0 port.
For using a FIFO the code I put together using the help files is this:
FUNCTION FifoScanning() InitHardware() variable/G EventCount EventCount=0 NewPath/O/M="Pick folder to save data" SaveDataPath NewFIFO ScanData // makes new FIFO NewFIFOChan /D ScanData, chan0, 0, 1, -10, 10, "V" NewFIFOChan /D ScanData, chan1, 0, 1, -10, 10, "V" NewFIFOChan /D ScanData, chan2, 0, 1, -10, 10, "V" //creates the channels to be scanned CtrlFIFO ScanData, deltaT=0.001 // sets the frequency of scanning CtrlFIFO ScanData, start //starts the FIFO; necessary to scan data into it -> scanning only works if FIFO is started first DAQmx_Scan /Dev="dev1" /TRIG={"/Dev1/PFI0"} FIFO="ScanData; 0/RSE; 1/RSE; 2/RSE;" //Starts data aquisition of the designated channels make/O /N=10000000 Wave0 //creates the counting variable needed to sort the waves according to the trial number FIFO2Wave ScanData, chan2, Wave0 //saved the data from chan0 to the Wave0 //wave must be created/designated first!! END
I tried to run it but it never even registers the trigger.
If there's any way to use the trigger to start and stop a wave-based scanning function I'll also gladly take this option.
Sorry for the long post; I'll gladly take any suggestions as to what might be wrong.
You can get what I presume is the desired behavior by moving Make and FIFO2Wave into a separate function, and then use the EOSH hook command to run that function.
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
August 10, 2015 at 02:46 pm - Permalink
Thanks for pointing that out! I completely overlooked this.
It took a while but we managed to adjust the code accordingly and get it to do what we want:
Not too pretty but it works.
Currently I've physically connected the trigger channel (Dev1/PFI0) and a free analog port (AI15) so I can check the readings from this port and terminate whenever the trigger turns off. Is there a more direct way of using the original trigger to accomplish this instead of continuously writing the FIFO values to a wave?
August 13, 2015 at 05:04 am - Permalink
I suppose what you would like would be a callback (like the EOSH flag) that would run your function when that signal changes state. Possible technically possible for me to implement in some future version of NIDAQ Tools MX, but not available now. If your solution works acceptably, I think you've got a clever solution.
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
August 13, 2015 at 09:43 am - Permalink
Precisely! It seemed so straight forward to have a native stop-trigger in addition to the start-trigger that it took me a while to figure out a way of essentially faking one.
But as you said: it works, so I'm perfectly content. From the forum-searches it also looks like this feature isn't missed by too many people.
Again, thanks a lot for the quick answers and pointing me to the right solution!!
August 13, 2015 at 09:50 am - Permalink
It seems as if the /EOSH-flag is of no consequence: after calling the DAQmx_Scan Command Igor immediately returns to whatever function is called next.
The scanning itself only starts once the trigger comes in but after the scanning is stopped Igor also proceeds with whatever function comes after it regardless of what is specified by the EOSH-flag.
For the DAQmx-DIO_Config-Function the help file states that the /EOSH-flag is only used if the values are scanned into Waves and not into FIFOs as FIFOs don't really have an end. Is this possibly also true for the DAQmx_Scan-Function?
August 21, 2015 at 08:44 am - Permalink
Using the /EOSH flag does not affect the flow of the program, it only invokes a command when scanning is finished, however that happens. If you use /BKG or you scan into a FIFO, the actual acquisition occurs in the background and the DAQmx_Scan operation returns without waiting for the scanning task to terminate, or even to start if you are using a trigger (technically, the task is started before DAQmx_Scan returns, but if you start it with a trigger it will wait for the trigger before actually taking data).
That's quite possible. I think that fDAQmx_ScanStop() does not cause the /EOSH hook command to be executed, and that's the only way to stop a FIFO scan. I would have to try it to be certain.
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
August 21, 2015 at 02:45 pm - Permalink
by now we finally tested the procedure and are now running into a new problem:
The trigger for the DAQmx_Scan command doesn't reliably evoke data aquisition. By now we have spaced the time interval between an instance of an off-trigger (i.e. the readings from the AI15 are below 2.5V) and the next on-trigger to 3 seconds or larger but it still keeps missing cues.
The code is nearly identical to the one I posted in August:
I'm starting to suspect that it might have something to do with the timing of generating the off-trigger, for example writing the data from the FIFO to the wave occurs at the same time as the trigger signal coming in and thus the rising edge is not detected.
If that is at all a possibility (and not just desperation on our part): is there another way of accessing the FIFO-data without impacting detection of the trigger?
I also tried setting the scan up using analog scanning to exploit minor fluctiations in the signal voltage to create the illusion of multiple start triggers comming in continuosly but this only produces error messages claiming analog triggering is not available. According to NI both the PCI-card and the DAQ board support analog triggering so I suspect there might be something off with the way I set it up?
Weirdly enought the off-trigger is always registered without fail. Would it be possible to setup FIFO-Scanning to start immediately after the function was called as well as have 2 instances of FIFO-scanning running in parallel?
The reasoning would be to keep monitoring the AI15 port using FIFO-scanning and immediate analysis and use this to start and stop the second FIFO-scan.
Right now I'm loosing about 10-20% of the data I'm interested in due to this so if there is any way of getting this fixed or at least some way of working around it I'd appreciate any ideas.
Thanks a lot
Janine
December 9, 2015 at 12:42 pm - Permalink
I don't know analog triggering well enough to have a firm answer...
I know that the APFI inputs have some restrictions, and aren't as sensitive as a regular analog input.
At least some devices allow you to use the first analog input channel in your scan list.
What is the full text of the error message?
What's connected to PFI0? Three seconds seems like plenty of time for what you're doing, unless you have huge amounts of data to move into waves.
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
December 9, 2015 at 03:32 pm - Permalink
thanks a lot for replying so quickly!
Depending on what type of Analog triggering I try to use I get different ones. Using Analog window triggering (
/TRIG={"/dev1/APFI0",3 ,1 ,5.085 ,5.09 }
), I get the following:Using Analog level triggering (
/TRIG={"/dev1/APFI0",2 ,1 ,5.085 ,5.09 }
), I get the following:If I supply the first scanned port as
/TRIG={"/dev1/ai0",2 ,1 ,5.085 ,5.09 }
I get the same error messages.I take it this means I can't use analog triggering at all but I'm unsure if this is because it won't accept the ports I supply or because I supplied the ports in the wrong format or because analog inputs are in general not accepted for some other reason.
The trigger to PFIO comes from a digital port (P0.0) on another BNC board (same BNC2090 version). For causing this trigger I use a simple fDAQmx_DIO_write() command to set this port to 1. This port is physically connected to the PFI0 port and the AI15-BNC port on th BNC port I'm running this procedure on.
I also started logging the time that passes between stopping the Scan and finishing the Save-command. This is in the range of 0.4sec at the most (for most it is around 0.15sec) and the final saved file is 3126KB in size. Also I tried using even longer intervals up to 10secs and smaller files around 612KB (using shorter waves) with no improvement. I guess this means igor is definitely ready to receive the next tigger, I'm just at a loss as to why it sometimes won't.
December 10, 2015 at 01:54 am - Permalink
That sure sounds like a noisy signal. Wiggle everything, make sure the connections are good and tight.
I can't tell much from the 2090 user manual. I wonder if they expect a pull-down or pull-up resistor somewhere. That's the older version of the connector block, designed for older devices. Some of those devices had open-collector digital outputs that required a pull-up resistor.
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com Those error messages both mean that your device (contrary to your expectations) does not support any type of analog trigger. Sometimes the NI specs are hard to read...
December 10, 2015 at 01:18 pm - Permalink