
Optimizing curving fitting for speed
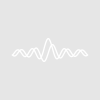
wholmgren
f(x) = Integral( g(x,v)p(v) dv)
The fitting function was originally written using the point-by-point method and a for loop to numerically integrate over v. I sped up the fitting by rewriting the code to use the all-at-once routine, but I still have the slow and imprecise for loop. I recently stumbled onto the Integrate1D function and thought that it might provide further speed/precision enhancements, but I'd like to know if anybody has any experience using it with curve fitting. I did see from a previous post that it looks like I'd have to use global variables to get the fit parameters into the integrate function.
Any other ideas would also be appreciated. Thanks.
You are correct that globals are required to pass fit coefficient to Integrate1D.
The fastest possible solution would be to write the function in C using the XOP Toolkit.
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
July 7, 2008 at 12:55 pm - Permalink
There were (4000-1000)/50=60 steps in the for loop. The help for integrate1D is slightly unclear as to what, exactly, the counts parameters is but it sounds like I should set it to 60 to achieve the same precision as the for loop. So, the integrate1D version is this:
Am I doing something wrong with the Integrate1D function? My guess is that in the for loop we can compute the integral for all points in the output wave basically simultaneously, but with the Integrate1D method the integral must be computed separately for each point. Thanks.
July 14, 2008 at 05:16 pm - Permalink
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
July 16, 2008 at 11:11 am - Permalink