
Pre-pend a zero to single digit number strings?
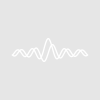
dcnicholls
I'd like to have the string read eg "07" instead of "7". Is there a way to format the string "numbers" so that 0 though 9 have a zero pre-pended but 10 through 59 don't?
So the following csv etries: 7,6,23.5 would be assemble as the string "07:06:23.5" (in other words, hours:mins:secs or degrees:mins:secs format)
At present the assembly I'm building reads "7:6:23.5"
Thanks
DN
prints the number vNum with a leading zero. when using string variables you could use sprintf instead of num2str like
where now the string sString is the string representation of the single-digit number vNum with a leading zero and a two-digit number with no leading zero appended.
gregor
March 22, 2010 at 06:15 am - Permalink
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
March 23, 2010 at 05:10 pm - Permalink
Both "%02d" (use 2 digits with leading zeros) and "%.2d" (print two digits of precision) give the desired result:
I would handle the fractional part separately. Here is my attempt:
March 23, 2010 at 06:38 pm - Permalink