
Problem with open files
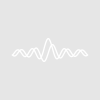
baroques_solari
So I have a bunch of folders (say, folder0, folder1, etc), in each folder there're a bunch of files (say file0, file1, etc). Here's a procedure that tries to load files in the folder specified by the user:
Function BatchLoad() String/G WorkingPath String RF Variable i, j GetFileFolderInfo/D/Q If (V_isFolder==1) WorkingPath = S_Path Newpath/O/Q SmbPth_WorkingPath, WorkingPath Endif // Do a bunch of things j = 0 String FileList = IndexedFile(SmbPth_WorkingPath, -1, "????") Make/O/N=(0)/T FileList_dump For (i=0; i<ItemsInList(FileList); i+=1) If (StrSearch(StringFromList(i, FileList), "dump", 0) != -1) Redimension/N=(1 + DimSize(FileList_dump,0)) FileList_dump FileList_dump[j] = StringFromList(i, FileList) Load_dump(j, RF) j = j + 1 Endif Endfor End
And here's the procedure to load individual file:
Function Load_dump(FileIndex, RF) Variable FileIndex String RF Wave/T FileList_dump String FileName = FileList_dump[FileIndex] Variable numRef, LineIndex, j String FileContent String/G WorkingPath Open/R/P=WorkingPath numRef as FileName // Do a bunch of things End
As I mentioned, I have a bunch of folders: folder0, folder1, etc. Everything worked perfectly well for folder0, but when i run BatchLoad() and specify to work on folder1 and other folders, it seems the Load_dump(FileIndex, RF) cannot correctly open files. Hope someone could take a look at and help me out. Thank you very much!
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
April 25, 2016 at 05:20 am - Permalink
This is identical to the same code with the first line removed. The second line uses a symbolic path named WorkingPath. This is correct if that is the name of the symbolic path. In that case, you don't need the String/G line. If, on the other hand, you have a string variable named WorkingPath that contains the name of the symbolic path, then you must use /P=$WorkingPath.
Execute this:
More generally, you are using global variables too much. With rare exceptions, global variables should not be used to pass information from one function to another. Using global variables for this purpose makes it difficult to understand and test code because it introduces non-obvious dependencies. You should use parameters instead. Global variables should be used primarily when you need to remember something between invocations of functions.
Rewrite your Load_dump function to use NO global variables. Whatever information it needs must be passed to it via parameters. Once you have done that, it will be easy to test Load_dump by itself, by executing a command from the command line. This will allow you to confirm that it works correctly or fix it without having to worry about the correctness of the calling function.
Once you have Load_dump working by itself, you should rewrite BatchLoad to use rely on parameters rather than global variables as much as possible. Once you have done that, you can test BatchLoad from the command line, knowing that you have already verified Load_dump.
If, having done that, it still does not work, use the debugger to debug it. Execute this:
April 25, 2016 at 08:17 am - Permalink