
Programatically calculating average
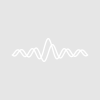
jmas340
Mon, 07/25/2016 - 01:09 pm
Function ButtonProc_average(ba2) : ButtonControl
Struct WMButtonAction &ba2
NVAR npoints1=root:npoints1
variable startindex,endindex
Switch( ba2.eventcode )
Case 2:
Prompt startindex, "First File Number"
Prompt endindex, "Last File Number"
KillWaves averagewave
Make/N=(npoints1) averagewave
Variable i
SVAR wavei
Wave testwave
For (i=startindex; i<=endindex; i+=1)
sprintf datai, "%s%d", "wave", i
averagewave = averagewave + datai
Endfor
Break
case -1:
break
EndSwitch
Return 0
Struct WMButtonAction &ba2
NVAR npoints1=root:npoints1
variable startindex,endindex
Switch( ba2.eventcode )
Case 2:
Prompt startindex, "First File Number"
Prompt endindex, "Last File Number"
KillWaves averagewave
Make/N=(npoints1) averagewave
Variable i
SVAR wavei
Wave testwave
For (i=startindex; i<=endindex; i+=1)
sprintf datai, "%s%d", "wave", i
averagewave = averagewave + datai
Endfor
Break
case -1:
break
EndSwitch
Return 0
When I hit the button, I get **a wave read gave error: Attempt to operate on a null (missing) wave
* Create a function that does what you want without a UI panel.
* Test and debug that function completely.
* Build a UI action call to that function.
So, you should code your function this way ...
NVAR npoints1=root:npoints1
variable startindex, endindex, ic
string wavei
Prompt startindex, "First File Number"
Prompt endindex, "Last File Number"
Make/O/N=(npoints1) averagewave=0
// possible method to average a set of waves
for (ic=startindex;ic<(endindex+1);ic+=1)
sprintf wavei, "wave%d", ic
wave thiswave = $wavei
WaveStats/Q/M=0 thiswave
averagewave[startindex-ic] = V_avg
endfor
return 0
end
Function ButtonProc_average(ba2) : ButtonControl
Struct WMButtonAction &ba2
switch( ba2.eventcode )
case 2:
DoMyAveraging()
break
case -1:
break
endswitch
return 0
end
Once you have written the function for DoMyAveraging and get it to work from the command line (type "DoMyAveraging()" on the command line), then your button call will work.
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
July 25, 2016 at 02:13 pm - Permalink
Just wanted to point out that there's already a pretty full-featured panel to average waves under the menu Analysis - Packages - Average Wave.
July 25, 2016 at 02:18 pm - Permalink
When I run something like what you say with the commands
WaveStats/Q/M=0 thiswave
I get an error that WaveStats gave error: expected wave name, even though I clearly declare it a line before.
July 26, 2016 at 07:32 am - Permalink
July 26, 2016 at 08:08 am - Permalink
The commands to average waves as you propose might be as such
duplicate/O wave0 waveave
waveave = 0
for (ic ...)
...
waveave += thiswave
endfor
waveave /= (endindex - startindex + 1)
Faster but less explicitly-intuitive versions of the code you want could eventually be designed with MatrixOp.
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
July 26, 2016 at 08:15 am - Permalink
I think you misunderstand the function of the "average waves" panel (sorry, I left off the "s" in my above comment). It does a point-by-point average of multiple waves, returning an averaged wave. What's more, you can programmatically call the underlying code by including the package and using the function fWaveAverage():
function easyAverage()
wave wave1,wave2,wave3
fWaveAverage("wave1;wave2;wave3", "", 0,0,"AveWave","")
end
You could programmatically make the list "wave1;wave2;wave3" to make the running average of whatever # suffixes you want. There's more detail on how to use all of this on the "Help" button in the Waves Average panel.
July 26, 2016 at 10:06 am - Permalink
You can use the Average Waves code to do this. If you include the panel in your procedure you can make a call to it from your code. You can do this as many times as you need. The advantage is that this is one line and the WaveMetrics code is bomb proof compared to something you will write yourself.
July 26, 2016 at 10:21 am - Permalink