
Programatically creating a polygonal ROI mask
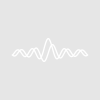
kpantzas
I'm looking to analyze data in an image locally between subsets of points of a sparse grid that I have computed for the image. I was reading through the IGOR documentation and thought of creating a ROI mask from a polygon whose vertices have been picked from the sparse grid and then using ImageGenerateROIMask to create the mask. The only problem with this idea is that for each subset of data I want to perform an analysis on I will need to display the image, the polygon, and run ImageGenerateROIMask, something that needlessly slows down the computation.
So my question is, how can I do all that without going through displaying the image with the new polygon every time I go from one polygon to the next?
The ROI wave needs to satisfy two conditions: it has to have the same dimensions as the image and it has to be unsigned byte wave. You can generate the wave using commands, e.g.,
A.G.
WaveMetrics, Inc.
February 18, 2016 at 11:50 am - Permalink
In the case I'm looking into the vertices do not form a rectangle; it's more like (20,30) (10,50) (40,20) and (50,80). That is why I was looking into drawing tools and how to programmatically define a polygon through the coordinates of it's vertices, then passing that shape to ImageGenerateROIMask, which I gather is how the ROI tool of the ImageProcessing toolbox does things.
Any suggestions as to how do that? If it's possible through a simple wave assignment statement I'm more than happy to use that.
February 19, 2016 at 02:04 am - Permalink
It sounds like you know the coordinated of the vertices of your polygon. So this method should be appropriate for you. Once you have created the XY waves, use
ImageBoundaryToMask
to create the ROI.February 19, 2016 at 05:43 am - Permalink
I ended up implementing the even-odd rule to test whether a point is in the polygon defined by the vertices and to set the value of the mask accordingly. Here's the test (adapted from https://www.ecse.rpi.edu/Homepages/wrf/Research/Short_Notes/pnpoly.html). It might not be the most elegant implementation, but it works nicely.
February 22, 2016 at 02:13 am - Permalink