
programmatic control of procedure window contents
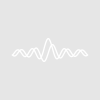
yamad
I would like to programmatically write to Procedure windows. Igor writes to the built-in procedure window when saving graph/window creation macros. Is there a function that allows a user-defined function to write to the procedure window (or auxiliary procedure windows)?
I was reading about writing to Notebooks using the Notebook operation and I thought I saw something about a function that allowed creation and writing to procedure windows as well. Am I mis-remembering? Note that for some of my use cases, writing to a text file and loading that file as a procedure will not work.
Thanks for the help.
You can write a file to disk and then load it as a procedure file. Execute this for help:
Usually this kind of trickery is a bad idea. It is very difficult to maintain or debug.
If you explain what your motivation is we may be able to suggest how to achieve it.
October 1, 2011 at 03:18 pm - Permalink
Ok, got it. It seemed to me that writing to a procedure from an IndependentModule might work, as long as the procedure being written to was not part of an IndependentModule. Oh well...
So, I actually have two goals:
1) I am writing a unit test framework for Igor (you can see my progress here: https://github.com/yamad/igorunit). To make writing tests non-painful, I am trying to implement a sort of preprocessor step that expands macros into valid Igor code. Unit test frameworks for C, like Unity and Check, use a similar approach. Right now, I have a python script that takes test descriptions from text files, preprocesses the tests and collects them in an *.ipf file. The python script then talks to Igor, asks it to include the test *.ipf, run the tests, and give the results back to python. This works fine right now, but I was trying to play with implementing the preprocessor step in Igor itself. It makes life slightly easier if I can write directly to a new procedure window. But as you say, creating a text file on disk should work too.
2) I also have implemented an emacs mode for Igor. The emacs editor, for me, makes me much much more productive than working directly in Igor. As has been mentioned elsewhere on the forum, Igor's code editor is pretty limited and so I am trying to break out of the Igor editor. The limitations of using an external editor are that 1) Igor locks the procedure file once it has been included, and 2) it requires all procedure code for an experiment to be held in external procedure files (that is, I can't edit built-in procedure code with emacs). Some people in our lab are quite averse to using external procedure files. Being able to break in and out of Igor with the built-in code (think of the It's All Text plugin for Firefox https://addons.mozilla.org/en-US/firefox/addon/its-all-text/) would solve both problems.
October 1, 2011 at 03:57 pm - Permalink
Yes, I think that is right, but Igor doesn't let you write to the procedure window.
I can see that you know what you're doing so you have my blessing to use trickery :)
I think you will have to generate a .ipf file on disk, either by writing a notebook to disk or writing directly using Open/FBinWrite or fPrintf/Close, and then loading the file using Execute/P INSERTINCLUDE or Execute/P LOADFILE followed by Execute/P COMPILEPROCEDURES.
October 1, 2011 at 05:03 pm - Permalink
Could you share with us your emacs-mode code?
October 4, 2011 at 02:03 pm - Permalink
It works by writing the commands to a procedure file and seeing if it compiles.
October 4, 2011 at 03:43 pm - Permalink
Is your primary use for this method to test new code without disrupting the compilation state of existing code or is it something else? I could see writing new code in a notebook and then testing by your method. Have you considered using a notebook as the front end to your function? Maybe you do this already.
Jeff
October 5, 2011 at 05:50 am - Permalink
Andy, I am also interested in what you use that code for. I had been considering using notebooks as the "source files" for generating procedures, but I haven't had the time to fully flesh out that idea.
October 5, 2011 at 03:16 pm - Permalink
October 5, 2011 at 03:55 pm - Permalink
The code _does_ disrupt the compilation state of existing code (unless it's an independent module). If the new code doesn't compile then the compiler will complain and tell you what didn't work. But then the code unincludes the file anyway.
THe main purpose of this method is to see if all the entries in a textwave would be syntactically correct if you wanted to execute it from the command line.
October 5, 2011 at 03:59 pm - Permalink
It's do-able, but complicated. See the Function Grapher package: Analysis->Packages->FunctionGrapher.ipf. In the procedure file, search for Execute/P.
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
October 5, 2011 at 04:17 pm - Permalink
Thank you.
October 6, 2011 at 07:30 am - Permalink