
Programmatically creating waves
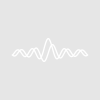
Cory K
One piece of code that I am having trouble with is programmatically creating waves.
In my procedure, heres what I have so far:
Make/N=200 'WaveA','WaveB','WaveC'..... etc
Prompt xWave, "Enter wave for x values : "
Prompt yWave, "Enter wave for y values: "
DOPrompt "Enter x and y waves", xWave, yWave
Is this correct for picking out two of the created waves, so later in the macro I can graph the two?
Also, is it possible to provide a list of the created waves within the prompt so the user can just pick 2 out?
Thanks,
Cory
November 14, 2008 at 01:44 pm - Permalink
For an explanation of the use of $, execute this:
DisplayHelpTopic "Converting a String into a Reference Using $"
As part of coming up the learning curve in programming, read manual chapters IV-1, IV-2 and IV-3.
November 15, 2008 at 06:46 pm - Permalink
Eg.
Make /O /N=200 'Wave A (units)', 'Wave B (units)'
or is that not necessary
November 17, 2008 at 02:10 pm - Permalink
How can I prompt a user to enter a file name?
I guess they could just type it in, but chances are, most users don't know the full pathname.
Is there a way to make a prompt with a 'Browse' button?
I would like to read each column of the file and programmatically assign the wave names.
November 17, 2008 at 02:24 pm - Permalink
Use the Open operation with the /D and /R flags. This presents a standard File open dialog and then your function that calls Open can get the full path of the file the user selected by inspecting the S_fileName string that the Open operation creates. The examples at the bottom of the command help for the Open operation should help you figure out exactly how to use it.
November 17, 2008 at 03:21 pm - Permalink
Alternately, you can create a panel that contains all of the prompts on it you want, including a "Browse" button which would call the Open operation. Making your own panel gives you many more options for interacting with the user, but is much more difficult to program. Your best bet might be to call the Open operation either before or after you call DoPrompt.
November 17, 2008 at 03:25 pm - Permalink
For some reason, either nothing is reading or I am not correctly using the data once it is read.
Can someone see where there is a problem or what I should add?
variable refNum
String xName ="", yName="", pathName="", fileName="", str=""
Open D/R =2/P =$pathName refNum as fileName
Variable err = V_flag
String fullPath = S_fileName
Prompt xName, "Enter wave for x values : ", popup WaveList("*", ";", "")
Prompt yName, "Enter wave for y values : ", popup WaveList("*", ";", "")
DoPrompt "Enter x and y waves", xName, yName
Wave xWave = $xName
Wave yWave = $yName
Display yWave vs xWave
November 18, 2008 at 11:51 am - Permalink
You are missing a slash before your D flag in the call to the Open operation. If that doesn't work, then I'd add a "print S_fileName" line after you call Open, to see if that string is getting set properly.
November 18, 2008 at 12:05 pm - Permalink
I added the slash before the D flag, and get an error.
When I go to compile the procedure, it highlights the 'R' and says:
"Ill-formed name"
This is what I typed :
Open /D /R =2/P =$pathName refNum as fileName
is that the correct syntax?
November 18, 2008 at 12:28 pm - Permalink
Is that why Igor is getting confused. Maybe I should be calling the function in a different way.
November 18, 2008 at 12:32 pm - Permalink
The /R flag doesn't take a value, so /R=2 is not valid. You probably want
November 18, 2008 at 04:59 pm - Permalink
Open is an operation, not a function, so doesn't directly return a value. To quote from Getting Started.ihf:
In the procedure window, operations are colored blue-green, and functions are colored brown, assuming you haven overridden the default colors.
November 18, 2008 at 05:05 pm - Permalink
That did the trick. Thanks.
For some reason I keep getting an error saying:
Error in user function, attempt to use null string variable
I cant seem to find which one it is talking about. Is there a debugger in Igor that will show me where the error occured?
November 19, 2008 at 02:15 pm - Permalink
Once I have the file name, I would like to do the following:
- Read the file (tab delimited text file)
- create a new wave for each column, each wave having a predetermined name
...Then the rest I can handle from there.
Thanks
November 19, 2008 at 02:21 pm - Permalink
My guess is that you are trying to use the S_fileName string in your function when it has not been defined, as is the case if the user clicks the cancel button in the Open file dialog box. I believe the command help for the Open operation gives an example that properly checks to make sure the cancel button hasn't been pressed.
Also, Igor does have a step debugger. From the command line, execute:
This will give you the run down on how to use the debugger. You might also want to enable NVAR/SVAR/WAVE checking after you enable the debugger, as that will cause the debugger to be activated when your code attempts to use a null string variable.
November 19, 2008 at 02:34 pm - Permalink
First of all, it's probably best if you create new threads for different questions, because otherwise the thread gets pretty confusing.
If you haven't already, I would suggest you take a look at the Igor manual for details on how to do what you want to do.
From the Igor command line, execute:
I suspect that your question will be answered in that section. Igor has a lot of built in capabilities for loading delimited text files.
November 19, 2008 at 02:37 pm - Permalink
I used the following from Igor's help files:
"Open /R/D/P = $pathName refNum as fileName"
where pathName and fileName are strings, and refNum is a variable
The error says that I am calling an empty variable during this line of code
After using print statements, I found that:
refNum = 0
but S_fileName is correctly displaying the selected file.
How can this be? I guess I am not understanding this part of the Open function:
"refNum as fileName" what exactly does that do
November 19, 2008 at 02:51 pm - Permalink
Thanks for your help
November 19, 2008 at 04:44 pm - Permalink
Just a thought .... have you looked at the file loaders already posted here? Perhaps you are re-inventing the wheel. In particular, the tab delimited file loader found here or as a part of the core package here might be useful.
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
November 19, 2008 at 05:05 pm - Permalink
Apart from the missing slash before the D, there is no real use for it if you actually want to read the file: just /R is readonly access.
/P=$pathname also does nothing as you have just set pathname to "" in the line above.
similarly the 'as filename' does nothing as you have also just set filename to "" above.
If you know the format of the input file, then LoadWave can do all of the loading of tab-separated files for you in one command, including putting up the dialog to find the file:
displayHelpTopic "Loadwave"
It's a very big help topic, but the operation can load a wide range of formats ,with subtle tweaks if needed.
LoadWave/J will load tab-delimited files, and LoadWave/J/L={...} where {...} are your preferred values for name lines etc. if known. This will save you being repeatedly asked for wave information that can be predicetd in advance. The are heaps of other tweaks and options to suit most delimited and general inputs.
Regards,
Patrick.
November 20, 2008 at 09:47 pm - Permalink