
Quickly get the mean of a wave with NaN values
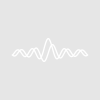
bsumlin
In python, I can do
average_despite_NaN = numpy.nanmean(data)
One line, no problem. In Igor, the mean() command says "If any values in the point range are NaN, mean returns NaN."
I need to quickly compare average values of several waves, each with random NaN values. In the data browser, I can look at the wave and it will tell me its average, even though there are NaNs. How come the mean() function can't do that, and what's the proper function?
WaveStats will ignore NaN values when calculating the average (stored in the V_avg output variable). For more information, execute:
DisplayHelpTopic "WaveStats"
If you only need the mean (and/or a few other statistics) you can use the /M=1 flag. That will make the calculation faster.
September 16, 2020 at 03:11 pm - Permalink
I would use
which gives you the expected result of three and is just one line. The nice thing about matrixOP is that it is composable, so calcuatling the max/min/sin etc. can be done with the same approach.
One caveat: This requires the current beta version of Igor Pro 9.
September 25, 2020 at 06:50 am - Permalink
Here is a (somewhat convoluted) one-liner doing the same, should you still be stuck with Igor 8:
This MatrixOp line calculates the sum of your wave without the NaNs and than divides it with the number of all non-NaN elements, which gives the mean.
September 25, 2020 at 10:01 am - Permalink