
Relative folder paths
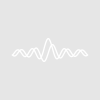
Irvine_audit
I am doing it like this right now:
Function relative_path() string prefix NewDataFolder root:Test NewDataFolder root:Test:Test1 NewDataFolder root:Test:Test1:Test2 SetDataFolder root:Test:Test1:Test2 DFREF dfr=GetDataFolderDFR() SetDatafolder ::: prefix=GetDataFolder(0) SetDataFolder dfr print prefix end
There must be an easier way to avoid messing with the
SetDataFolder
operation. I would love to have a solution where I can store the name of a folder relative to the current folder with like one command.If I want to use the string-related commands like
::GetDataFolder(0)
I get told that I cannot access a non-existent data folder even if I assign it to a string first. It is all quite confusing to me.Can anyone help me?
outputs
root:A:C: root:A:B:
December 3, 2015 at 02:22 am - Permalink
I thought there might be something where I can avoid the whole "SetDataFolder" operation altogether.
But I just realized that it is probably inherently not possibly to just access the foldername of a parent folder withouht setting it first.
Something like this will not work ("Tried to access parent folder of root folder or a non-existent data folder"), although I do not really understand why.
By the way it does not matter whether it is
GetDataFolder(1)
or (0).I do understand that the possible results of
:::$path2
do not make sense to pass to a string, because without any folder operation it is not clear what is implied. But I do not understand the error message. As if Igor already checks the existence of folders at compile time?December 3, 2015 at 03:13 am - Permalink
Kurt
December 3, 2015 at 03:49 am - Permalink
December 3, 2015 at 05:46 am - Permalink
December 3, 2015 at 07:02 am - Permalink
RefPath_resolve
function inrefpathutils.ipf
will give you back a string containing the full object reference path. In your case, you can get the parent folder withRefPath_resolve("::")
. You can use it like:If I understand your goal correctly, this function was designed for your situation. You can specify reference paths as a string and you get back "the right" absolute path as a string. The path does not have to point to something that exists, so you can use it to create names for something you want to create:
January 28, 2016 at 01:20 pm - Permalink
Another GetDataFolder will give you the string value, if that's what you're interested in. I have this solution from another post here on Igor Exchange, but I don't remember which.
February 1, 2016 at 01:16 am - Permalink