
Replace NAN for V_avg
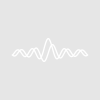
Turtle Sandwich
Function replace_nan(data_wave) Wave data_wave Variable i String destName = NameofWave(data_wave)[0,11]+"_modified" Make/O/N=(numpnts(data_Wave))/D $destName Wave/D dest = $destName dest = NaN Wavestats/q data_wave For (i=0; i<(numpnts(data_wave)); i+=1) If (data_wave[i]!=NaN) dest[i]=data_wave[i] Elseif (data_wave[i]== NaN) dest[i]=V_avg Endif Endfor appendtotable dest End
data_wave[i]!=NaN
anddata_wave[i]== NaN
, use thenumtype
function. That would give you this:Second, you don't need an explicit for loop here. Instead, you could use waveform assignment. Waveform assignment is much faster than explicit for loops. That leaves you with this:
Note that I also replaced the Make command with a simpler Duplicate command.
Third, in many cases, there's a way to use MatrixOp to give you improved performance and often simpler syntax. That's true in your case, giving you this:
March 3, 2016 at 08:17 am - Permalink
DisplayHelptopic "NumType"
.Instead of a loop you can use:
Check out
DisplayHelptopic "? :"
to see what is happening.EDIT: Too late ;-)
March 3, 2016 at 08:22 am - Permalink
and exploit the fact that comparing NaN to everything is false. But I must strongly support Adams suggestions, especially the
matrixOP
solution. Which is faster, better readable and shorter.March 3, 2016 at 09:08 am - Permalink
March 3, 2016 at 10:34 am - Permalink