
Replacing values
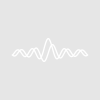
etd810
I'm trying to write a procedure in which I can replace some wind direction values from letters to their corresponding values in degrees. For example, I want to replace every time I get NE with the number 45. I'll like to make a new wave in which I have the converted values in degrees. So far I haven't been able to do so. I'll appreciate any help with this!
Here is a function that will return the appropriate number of degrees for a small number of direction names:
It is written so that it can be used in a wave assignment like this:
I leave it as an exercise for the reader to expand the selection of direction names :)
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
July 31, 2014 at 03:18 pm - Permalink
What I tried before was an if statement, but it didn't work.
I've been trying to work with the procedure you posted, but I can't make it work. I don't have much experience with the program.
Here is what I wrote. Hope you can help me figure out what I'm doing wrong. When I try to use it it gives me an error that reads: "ambiguous wave point number".
August 3, 2014 at 06:49 am - Permalink
to this:
p is the implicit loop variable for the wave assignment statement.
Igor does this indexing automatically for waves on the righthand side of an assignment statement but not for parameters to a function call.
For background information, execute:
August 3, 2014 at 07:46 am - Permalink
I tried the suggestion you posted, but I'm still getting the same "ambiguos wave point error" when I try to execute the function.
August 4, 2014 at 10:58 am - Permalink
Change this ...
... to this ...
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAHuntsville
August 4, 2014 at 11:16 am - Permalink
Execute Test() from the command line.
August 5, 2014 at 01:01 am - Permalink
However, this procedure is not working how I expected. It does create a new column, but only with the same information that the procedure has. It is not replacing my acquired wind direction data from letters to degrees, which my goal.
Hope you can help me with this!
August 6, 2014 at 04:03 pm - Permalink
newDegrees has the wind directions in degrees.
August 6, 2014 at 04:22 pm - Permalink
Are you saying that you want to start with a text wave with direction names, and end up with the same wave, but with numbers of degrees? You can't directly convert a text wave into a numeric wave. You could put strings that represent numbers into your original text wave, but then you can't use it for computation. Here is an example that results in numbers-as-text:
But as I said, I don't know why you would want to do that.
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
August 7, 2014 at 01:10 pm - Permalink
Yes, that is what I want to do. I want to have two waves, one in which I have my original wind directions in letters (NE, E, etc), lets say wave0, and a new wave in which these wind directions in letters are replaced by their corresponding degrees values (wave1). So every time I get a NE in wave0 i get 45 in wave 1 and when I get E in wave0 I get 90 in wave1 and so on. This way they can be graphed in a 2D line plot alongside other data. I often do this manually, but it can be very time-consuming when you have to do it with a bunch of files.
August 9, 2014 at 06:28 pm - Permalink
Those commands to be executed on Igor's command line were an example to show you how to use my function. The first Make command makes an example text wave with direction names in it. The line after that fills it up with example direction names. The third command (second Make command) makes a numeric wave of the same length as the text wave. It will receive the numeric wind direction in degrees. The fourth line is a wave assignment that uses the function to fill the second, numeric, wave with degrees based on the names in the first, text, wave.
You need to put the function definition itself in a procedure window. The main Procedure window (Windows menu->Procedure Windows->Procedure Window) will do the trick. The other lines are to be executed on Igor's command line, type them below the red line.
Does that help?
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
August 11, 2014 at 10:37 am - Permalink
The problem was that I was adding the make commands in the procedure and for some reason they don't work when I put them in the procedure (even in a separate function), but work when I type them manually.
Thank a lot for your help!!
August 11, 2014 at 06:18 pm - Permalink
And since the function itself was written to take in one value and return one value, putting wave assignments inside that function won't work. But you can wrap up the commands (which I only intended as an example of how it all works) in a second function. That function might make a new numeric wave of the right size and then execute the wave assignment:
That Make command does a lot: it makes a new wave, of course, but it does it by taking the name of the input wave and appending "_Deg" to the name. The $ tells igor that what follows is a string expression, and that string expression should be evaluated and the result used as the wave name. The final flag /WAVE creates a local symbolic name for the new wave to be used in the code that follows. That is necessary because when the function is compiled, we have no idea what the name will be when the function is run.
It also used the numpnts() function to make sure the new numeric wave has the same number of points as the input text wave.
The second line (the wave assignment) implies a loop over all the points in outwave. On the right side, Igor arranges to take each corresponding point from windDirNames and feeds them one at a time to GenWindDirection(). The result of GenWindDirection() is put into the currently active point in outwave.
Now, for testing and as an example, on the command line make a new text wave with wind direction names:
make/n=16/T WindDirNames={"NNE","ENE", "ESE", "SSE", "SSW", "WSW", "WNW", "NNW", "NE", "SE", "SW", "NW", "N", "E", "S", "W"}
Now that you have an example of a text wave with direction names, now use the second function to make a new numeric wave with degrees:
NumericWindDirWave(WindDirNames)
Now bring up the Data Browser (Data->Data Browser) and you can confirm that you have a new wave called "WindDirNames_Deg" that is numeric. To see that it has done what you want, do this:
Edit WindDirNames, WindDirNames_Deg
If you haven't already (or maybe again, as a refresher) check out Help->Getting Started. Do at least the first half of the Guided Tour.
You may also want to read about wave assignments:
DisplayHelpTopic "Waveform Arithmetic and Assignment"
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
August 12, 2014 at 09:40 am - Permalink
Thanks a lot for your help!
August 14, 2014 at 01:07 pm - Permalink