
simple color mixing
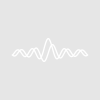
chriswo
I want to more or less automatically colorize my traces in any given plot using a small program:
The idea is just to mix the rgb values in equidistant steps, similar to the "make traces different" package, however with selfdefined colors (two at the moment).
The code appears to be ok, as far as I can tell, but i don't understand, why it won't work (i.e. it colorizes all traces black)
I tried it with and do-while loop too, wasn't succesful either, can you guys please help?
function mixcolors(c1r,c1g,c1b,c2r,c2g,c2b) variable c1r,c1g,c1b,c2r,c2g,c2b variable i i=0 // count the number of traces in the graph string names=tracenamelist("",";",1),name variable count=itemsinlist(names) //iterate through the traces and change their colors for(i=0;i<count;i+=1) ModifyGraph/Z rgb[i]=(round(abs(c1r-i*(c1r-c2r)/count)),round(abs(c1g-i*(c1g-c2g)/count)),round(abs(c1b-i*(c1b-c2b)/count))) endfor end
I plan to implement a hex2rgb converter once this works to get rid of the 6 input variables, but that's a different story.
rgb[i]
, the i value is an integer. You need to create a string and take the tracename from the list and put that there. Something like (untested):June 4, 2015 at 02:10 am - Permalink
Actually for completeness, I've worked it out in the meanwhile like this:
June 4, 2015 at 04:01 am - Permalink
FWIW: equal distances in RGB color-space are not equal distances in terms of human color perception. For that, you need to work in Lab color space.
I am not aware of a good general solution for picking up 'count' most distinct colors for a graph (even is you assume a starting color say c1). My own preference is to use HSL color-space. For small 'count' I simply divide the hue circle in equal intervals.
A.G.
June 4, 2015 at 07:29 am - Permalink
and run it with
modifygraph
as follows:RGB($ExpName)=(HSL2RGB(i*210/NumExp+50, 240, 100, 0), HSL2RGB(i*210/NumExp+50, 240, 100, 1), HSL2RGB(i*210/NumExp+50, 240, 100, 2))
also have a look at the "Make Traces Different" package hidden in the "Graph/Packages" menu.
HJ
June 4, 2015 at 07:59 am - Permalink