
Sort DataFolders using GetIndexedObjName or GetIndexedObjNameDFR
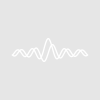
BMangum
In this case the order of the waves on the table matters.
In case it helps, I am using liberal folder names.
When I created the folders, I was not super careful about the order I did them.
When I run the bit of code below, I build a table with the waves in the order of the folder creation date.
This is undesirable behavior, I want them sorted alphanumerically.
I can toggle the preferences of the data browser to change the display order, but GetIndexedObjName seems to be insensitive to this fix.
Is there some way to re-order or sort the datafolders in conjunction with GetIndexedObjName such that I can get them in the desired order?
Edit Variable numfolders = CountObjects(tempname, 4) for (i=0; i< numfolders; i+=1) Tempfolder = tempname + "'" + GetIndexedObjName(tempname, 4, i) + "'" SetDataFolder tempfolder Appendtotable wave0 endfor
FYI - my folder names are:
2015_06_08
2015_06_06
2015_06_07
etc...
If you don't want to do that, this code shows how to achieve what you want.
June 9, 2015 at 12:40 pm - Permalink
Thanks, I was thinking maybe I was missing some setting or flag somewhere that would simplify this.
Apparently not.
The programmatic solution is the best one as there are a very large number of datafolders.
Thanks for the code snippet to achieve that!
June 9, 2015 at 01:34 pm - Permalink