
SVAR_Exists
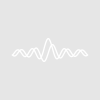
patg
string/G globalString="test string"; SVAR/Z gStr=$"globalString"; if(SVAR_Exists(gStr)) print gStr; endif; killStrings globalString; if(SVAR_Exists(gStr)) print "gStr: cannot be true..."; endif;
The second print will cause an error. Any way around this?
SVAR/Z gStr=$"globalString"
assignment before the second test. When I checked, Igor didn't complain about this, but it seems that it could get unwieldy, depending on your code.
I also thought to try testing if the string was null using
if(numtype(strlen(gStr))==2)
but this line provoked the error "attempt to use a null string variable." This seems to qualify as a good working example of Catch-22.
July 3, 2014 at 05:18 am - Permalink
In fact like noted by jtigor, if you change your example code to
it works as you anticipated your code to work. But I am sure that some of the IGOR staff will have the correct answer, these are just my thoughts ...
--
Gregor Kladnik
ALOISA beamline @ Elettra synchrotron
July 3, 2014 at 05:54 am - Permalink
exists
command an test for 2.July 3, 2014 at 05:55 am - Permalink
For waves the corresponding code
does what you expect.
You can write a wrapper
and use it like
Although in principle I would not use global strings and variables a lot.
July 3, 2014 at 08:31 am - Permalink
At compile time, they signal to the compiler that a given name used in your code refers to a string variable, numeric variable or wave. That allows the compiler to create the correct kind of code to deal with the particular object.
At runtime, they signal Igor to actually look up the real object and store a pointer to the object in the NVAR, SVAR or WAVE variable. This look-up must happen at a time when the object actually exists (or in your case, when it doesn't exist). A common error in Igor coding is to assume that these things are just like C or C++ variable declarations, which have only a compile-time function. That causes people to put them too early in their code, before the objects are created. That leads to unexpected NULL variable or NULL wave error messages.
In your case, the first instance of SVAR connects gStr to the existing global string variable. Then you kill the variables. But gStr still has a pointer to the dead string, so SVAR_Exists() gives an apparently wrong answer. Repeating the SVAR statement after KillStrings re-loads gStr, and this time it will not find globalString, and SVAR_Exists() will return FALSE.
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
July 3, 2014 at 08:58 am - Permalink
July 3, 2014 at 08:50 pm - Permalink
Apologies for quoting myself.
July 4, 2014 at 07:27 am - Permalink
For the most part you can think of SVARs and NVARs behaving as normal variables but to be precise, they are *references* to variables.
There is a difference between a null string variable and a null reference:
In the case of str, the string exists but its value is NULL.
In the case of sv, the string does not exist.
strlen can handle an existing string whose value is NULL - it returns NaN.
strlen can not handle a non-existent reference to a string variable - it generates an "SVAR reference to string failed" error.
If there is a chance that an SVAR may not point to an existing string variable then you need to use SVAR_Exists.
As this thread illustrates the reference stored in an SVAR depends on the state of affairs at the time the SVAR statement executes. This is the the analogous to the problem of creating a WAVE reference before the wave exists:
July 4, 2014 at 09:23 am - Permalink