
Updating Panel instead of creating a new one
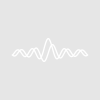
jmas340
Command(a,b)
And my procedure is
Macro Command(a,b) //bunch of stuff DataPanel() End Window DataPanel() : Panel PauseUpdate; Silent 1 NewPanel/W=(500,100,1200,600) as "Data" //stuff such as Display/W=(70,270,320,450)/HOST=# a
How can I do this so that when I send Command(a,b), it only updates, not creates a newpanel.
It depends on what aspect of the panel you want updated. If you have, for instance, a SetVariable control that needs an updated value, you would use the SetVariable command with the "value" keyword if you originally made it with value=_NUM:, or you would simply assign a new value to the global variable if your SetVariable is attached to a global variable.
What needs to be updated?
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
July 26, 2016 at 10:57 am - Permalink
July 26, 2016 at 11:13 am - Permalink
July 26, 2016 at 11:15 am - Permalink
* Learn to use Functions not Macros. I believe Macros are mostly considered obsolete except for backward compatibility.
* Learn to use DataFolderDFR functions to set or define locations of waves. These references to folders will help keep the code "transportable" as you move from one folder to another or one experiment to another and accidentally change the location of the current data folder.
* Put return values at the end of the Functions (e.g. return 0). This is good programming practice.
* Replace the Window PulseInfo() with a function call that is a bit more understandable, e.g. Function ShowPulseInfoPanel(). Again, good programming practice.
* Correspondingly remove all the unnecessary PauseUpdate, DelayUpdate, and Silent 1 commands in the ShowPulseInfoPanel() function. They are only there when the panel is being generated in a "one-step-at-a-time" approach as per a command line entree. They are useless in function calls.
Secondly, as for the question about updating graph displays, I have a suggestion and some observations.
* Suggestion: Display your graphs separately and debug their operation BEFORE you embed them in a panel. Graphs must operate independently of a panel anyway (as you have now learned).
* Observations: Your panel/graph display code has no definitions for the "$" waves that are to be displayed in the graphs. This means, they are basically non-existent references. Also, your Pulse code plays jostles around with "$" references to strings as wave names. This is confusing at best and potentially damage prone at worse. Any of these mistakes could leave the code "hanging" for lack of a proper reference point to wave, because that wave will not really exist at run time or it will exist somewhere in some location that is not the current data folder or it will not even be defined in the right way even when it does exist where it is supposed to exist.
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
July 26, 2016 at 11:42 am - Permalink
If you are creating new waves, use AppendToGraph to add them to your graph:
It will take a different function from the one that built the panel with the graphs. I have left some things that you will have to figure out. It is a good idea, when you create the panel to give it a name that isn't likely to be the same as someone else's name:
NewPanel/N=jmas340Panel ...
and then you can use a hardcoded name in the update function:
AppendToGraph/W=jmas340Panel#G0 ...
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
July 26, 2016 at 04:13 pm - Permalink